Every developer reaches a point where mastering the nuances of PHP becomes indispensable. Dive into this comprehensive guide to uncover a wealth of PHP tricks that will make your coding journey smoother and more efficient.
Explore the fundamental steps for getting started with PHP, discover essential functions that simplify complex tasks, and delve into advanced techniques that can elevate your coding skills. Learn how to optimize your PHP code for better performance and gain insights from real-world tips that experienced developers swear by.
Whether you're just beginning your PHP journey or looking to refine your existing skills, this article is packed with valuable information to help you navigate the dynamic world of PHP development.
- Getting Started with PHP
- Essential PHP Functions
- Advanced PHP Techniques
- Optimizing PHP Code
- Real-World PHP Tips
Getting Started with PHP
If you are new to PHP, you're stepping into a world of seamless server-side scripting that can transform your web development experience. PHP, which stands for Hypertext Preprocessor, is one of the most popular languages for web development. The language is open-source and especially suited for developing dynamic and interactive websites. Perhaps one of the most appealing aspects is how smoothly it integrates with HTML, enabling developers to embed PHP code directly within HTML files.
To begin your journey, the first thing you'll need is a local development environment. You can use tools like XAMPP or WampServer, which package Apache (web server), MySQL (database), and PHP together. This setup is essential since it mimics a live server environment on your local machine, allowing you to test your scripts before deploying them live. PHP development is versatile and can run on most operating systems like Windows, macOS, and Linux.
Once you've set up your local environment, your next step is writing your first PHP script. The simplest form of a PHP script starts with the <?php
tag and ends with ?>
. Everything in between is your PHP code. For instance, here's how you'd create a basic script that outputs 'Hello, World!':
"The future of PHP looks promising as it continues to evolve, meeting the needs of developers worldwide." - Zend Technologies
<?php
echo 'Hello, World!';
?>
Embedding this code in an HTML file allows you to mix HTML and PHP seamlessly. When this file is accessed through a browser, the server processes the PHP code and sends the resulting output, along with any other HTML, to the browser. This flexibility is one of the reasons why PHP remains so widely adopted.
Another important aspect of getting started with PHP is understanding its error reporting features. PHP provides robust mechanisms for error handling which, when properly configured, can save you from countless debugging headaches. You can control the error reporting level by setting the error_reporting
directive in your php.ini
file or within your script using the built-in error_reporting()
function. Setting an appropriate error reporting level aids in identifying problematic areas in your code early on.
Working with databases is a key part of PHP development. PHP supports a wide range of databases, including MySQL, PostgreSQL, SQLite, among others. The most common choice is MySQL due to its reliability and ease of use. You can connect to a MySQL database using the mysqli_connect()
function, or better yet, use PHP Data Objects (PDO) for a more secure and feature-rich approach. Here is an example of how to connect to a MySQL database using PDO:
<?php
try {
$pdo = new PDO('mysql:host=localhost;dbname=test', 'username', 'password');
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
echo 'Connected successfully';
} catch (PDOException $e) {
echo 'Connection failed: ' . $e->getMessage();
}
?>
This ensures a secure connection to your database while providing error handling capabilities. Mastering these basics lays a solid foundation for more advanced PHP tricks and techniques.
Essential PHP Functions
Diving into the world of PHP, there are some pivotal functions that every developer should have in their toolkit. These functions can help streamline coding, making your development process faster and more efficient. Understanding and utilizing these functions can truly be a game-changer.
One of the most commonly used functions is array_search(). This function searches for a specific value within an array and returns the corresponding key if the element is found. This is particularly useful when you need to locate data within large datasets. By using array_search(), you can save a significant amount of time and code.
Another indispensable function is array_map(). It allows you to apply a callback function to each element of an array. This is incredibly powerful when you need to modify each item in an array without writing loops manually. Imagine you have a list of numbers and need to square each one. Instead of creating a loop, you can apply the array_map() function for a quick solution.
Working with Strings
When it comes to string manipulation, PHP offers abundant functions to ease the process. For instance, str_replace() is frequently used to replace all occurrences of a search string with a replacement string. This function can be a lifesaver when dealing with template engines or user inputs that need sanitizing.
An often overlooked but highly useful function is substr_count(). It helps count the number of times a substring appears within a string. This can be beneficial for text analysis, particularly if you are working on applications that require natural language processing.
According to Rasmus Lerdorf, the creator of PHP, "The best way to learn any programming language is to read and dissect other people's code." By understanding and implementing such essential functions, you enhance your ability to build complex applications more efficiently.
Handling Data
When working with data, PHP makes it simple to handle JSON. The json_encode() and json_decode() functions are essential for developers working with APIs. These functions help convert data structures to JSON format and back, facilitating seamless data exchange between client and server.
Another noteworthy function is filter_var(). It is used to validate and sanitize data, which is crucial for security. Whether it's an email, URL, or IP address, this function can help ensure the data meets predefined criteria without opening up security vulnerabilities.
By incorporating these essential PHP functions into your workflow, you’ll find your coding experience to be much more dynamic and efficient. These tools, when used to their fullest potential, can significantly improve the quality of your development projects and make everyday coding tasks less cumbersome.
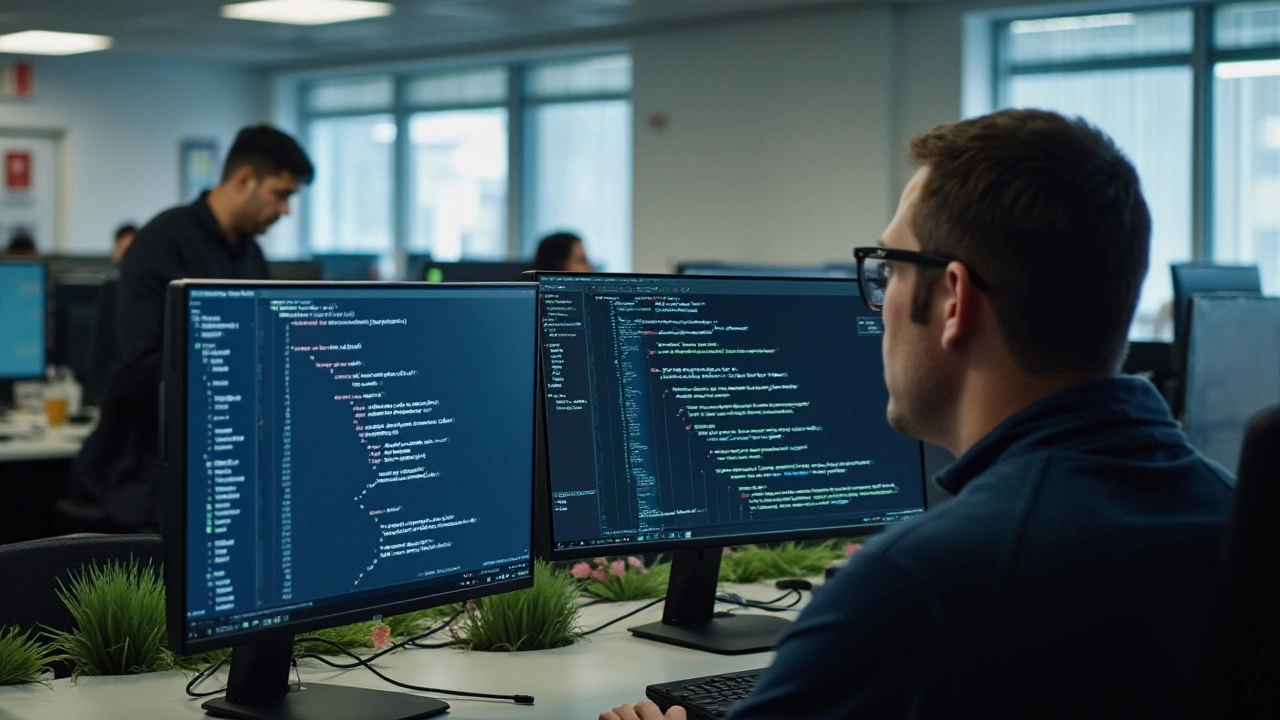
Advanced PHP Techniques
As you dive deeper into PHP development, you'll discover a treasure trove of advanced techniques that can enhance your coding efficiency and expand the functionality of your applications. These techniques ensure that your applications are not only robust but also optimized for performance.
One powerful technique involves using PHP's built-in functions to their full potential. Functions like array_map()
and array_reduce()
can dramatically reduce the amount of code you need to write. For example, array_map()
allows you to apply a callback function to each element of an array, effectively optimizing the processing of that array. Another indispensable function is array_filter()
, which can be used to filter out elements of an array that do not meet certain criteria.
Optimizing database interactions is crucial for performance. Implementing prepared statements is a must. They not only provide a safeguard against SQL injection attacks but also enhance execution speed by pre-compiling the SQL code. Using Object Relational Mapping (ORM) tools like Doctrine can also streamline your database operations, making your code cleaner and easier to maintain.
Another essential aspect is caching. Using tools like Memcached or Redis, you can store frequently accessed data in memory, significantly reducing the load on your database and speeding up your application. PHP supports multiple caching mechanisms, and choosing the right one can have a significant impact on your application's performance.
Moreover, adopting a full-stack PHP framework like Laravel can save you time and effort by providing a rich set of features that handle common tasks automatically. Laravel's Eloquent ORM makes it easy to interact with the database, and its Blade templating engine simplifies the process of rendering views. Using these built-in utilities can help you focus more on your application logic rather than the repetitive tasks.
Additionally, leveraging Composer, PHP’s dependency manager, can bring in a plethora of libraries and components that can be seamlessly integrated into your project. This not only speeds up development but also ensures that you are using well-maintained and reliable packages.
For debugging and logging, don't overlook the power of Xdebug. This tool allows you to set breakpoints and step through your code, making it easier to identify and resolve issues. Setting up Xdebug can be a game-changer when it comes to tracking down elusive bugs, and integrating it with an IDE like PHPStorm can further streamline the process.
Finally, consider integrating Continuous Integration/Continuous Deployment (CI/CD) pipelines into your development workflow. Tools like Jenkins or GitHub Actions automate the process of testing and deploying your code, ensuring that new features and fixes are rolled out efficiently. Embracing CI/CD practices can drastically reduce the time it takes to get new updates into production, boosting both productivity and reliability.
As Rasmus Lerdorf, the creator of PHP, once said, "I actually hate programming but I love solving problems."
Embracing these advanced PHP techniques will not only make your code more efficient but will also help you tackle complex problems with greater ease, ultimately making you a more effective programmer.
Optimizing PHP Code
Optimizing your PHP code is crucial for ensuring that your web applications run smoothly and efficiently. One of the first things to consider is minimizing the use of loops. Nested loops can often slow down performance, so it’s better to find alternative methods for tasks that would typically require such loops. Additionally, using built-in PHP functions wherever possible instead of custom-written code can greatly enhance speed and reduce complexity.
Another effective optimization technique involves using caching mechanisms like Memcached or Redis. These tools store frequently accessed data in memory, reducing the load on your PHP scripts and databases. For instance, caching the results of expensive database queries can save a significant amount of time and resources, especially for high-traffic websites.
An often overlooked aspect of PHP optimization is proper use of string functions. Functions like str_replace are much faster compared to regular expressions. Similarly, leveraging array functions can streamline your code, making it not only faster but also easier to read and maintain. This is where a good understanding of PHP’s array manipulation functions, such as array_map and array_filter, comes in handy.
Profiling your PHP code helps in identifying bottlenecks. Tools like Xdebug or Blackfire offer great insights into which parts of your code are consuming the most resources. Once you identify the slow sections, you can focus your optimization efforts more effectively.
Consider memory usage as well. In PHP, reference counting and garbage collection play a significant role. Knowing how to manage these can help make your code more efficient. For instance, unset variables that are no longer needed to free up memory. Use pass-by-reference where applicable to avoid unnecessary copying of large datasets.
Database interaction is another critical area for optimization. Using prepared statements not only secures your application but also enhances performance. They allow the SQL engine to cache the query plan, reducing the time spent on executing similar queries. Additionally, indexing your database columns speeds up queries significantly, as the database can quickly locate the rows that match the criteria.
"Optimizing your code isn’t just about improving speed—it’s about making your application scalable and maintainable," says Nikita Popov, a PHP core developer.
Lastly, always keep your PHP version updated. Each new release of PHP includes performance improvements and bug fixes that can make your code run faster. PHP 8, for instance, introduced the JIT (Just-In-Time) compiler, which can execute bytecode natively, resulting in significant performance gains.
Through these techniques, you can ensure your PHP code not only runs faster but is also more robust and maintainable. As with any programming language, understanding the intricacies and best practices can turn you from a good developer into a great one.
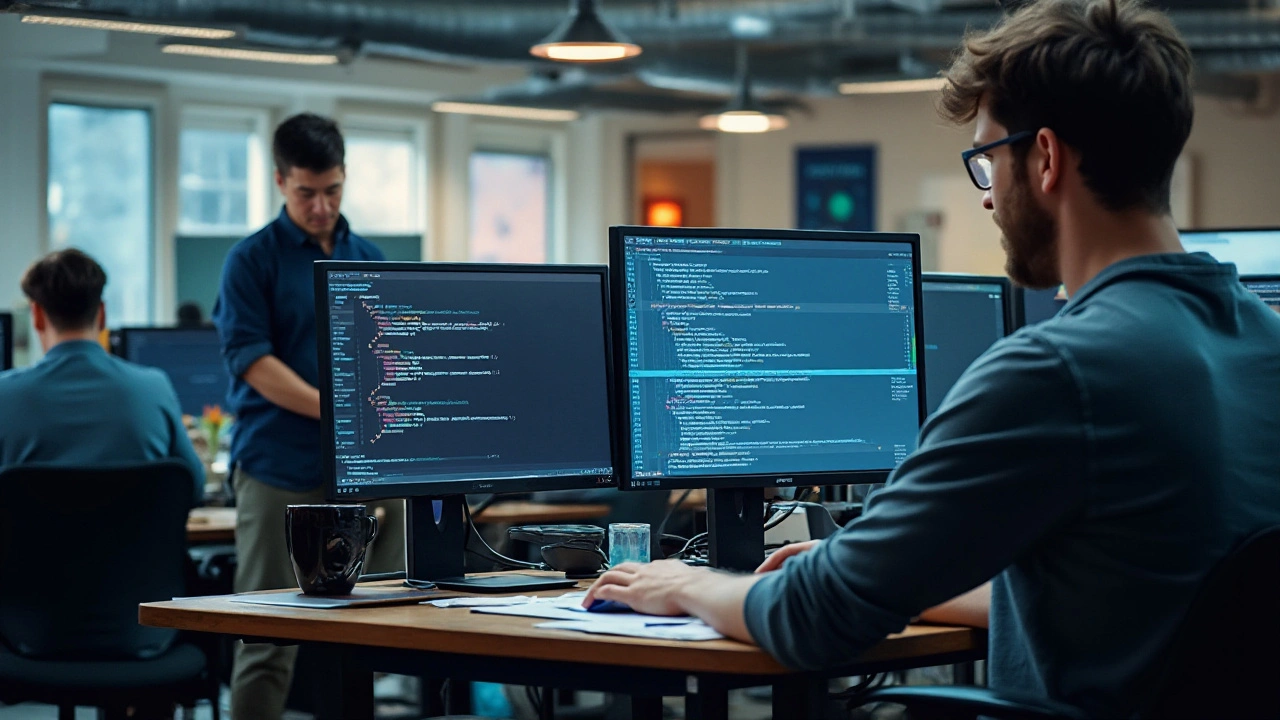
Real-World PHP Tips
When it comes to PHP development, there are countless tips and tricks that can make your life much easier. Here are some real-world PHP tips that every developer should know. These pointers not only improve your coding skills but also make the development process smoother and more efficient. For starters, always remember to keep your code clean and organized. A messy codebase is a nightmare to maintain and debug. Use consistent naming conventions and always comment your code. This makes it easier for you or anyone else to understand your code in the future.
Another important tip is to make use of PHP's built-in functions. PHP offers a plethora of built-in functions that can save you a lot of time and effort. For instance, instead of writing your own function to sort an array, you can use PHP's built-in sort() function. This not only saves time but also ensures that your code is more efficient and less prone to errors. It’s also a good habit to keep updating your PHP knowledge. New functions and features are added with each PHP release, and staying updated helps you write better and more secure code.
Security is a crucial aspect of any PHP application. Always sanitize and validate user input to prevent SQL injection, cross-site scripting (XSS), and other security vulnerabilities. Use prepared statements and parameterized queries to interact with the database. This is a simple yet effective way to prevent SQL injection attacks. Another security tip is to use HTTPS for all communications to ensure that data is transmitted securely.
When it comes to working with databases, a useful tip is to use ORM (Object-Relational Mapping) frameworks like Doctrine or Eloquent. These frameworks streamline the database interactions by allowing you to use PHP objects instead of writing raw SQL queries. This not only makes your code more readable but also less error-prone. Speaking of databases, always backup your databases regularly. Data loss can be catastrophic, and regular backups ensure that you can restore your data in case of any issues.
Another tip is to use version control systems like Git. Version control helps you keep track of changes in your codebase and collaborate more effectively with other developers. It’s also useful for reverting to previous versions of your code if something goes wrong. Learning and using Git can significantly improve your PHP development workflow.
Performance optimization is another crucial aspect of PHP development. One way to optimize your PHP code is by minimizing the use of loops. Loops can be very resource-intensive, especially nested loops. Try to find alternative ways to achieve the same result with fewer loops. Also, make use of caching techniques to improve the performance of your PHP applications. Tools like Memcached and Redis can help you cache data and reduce the load on your database.
Testing is another critical aspect of PHP development. Make use of PHPUnit, a popular PHP testing framework, to write unit tests for your code. Testing ensures that your code works as expected and helps you catch bugs early in the development process. It’s also a good practice to write automated tests to save time and ensure consistency.
Lastly, always keep learning and improving your skills. The tech world is constantly evolving, and staying updated with the latest trends and best practices is crucial. Join PHP communities, attend conferences, and read blogs to stay in the loop. As the saying goes, “The more you learn, the more you realize how much you don't know.” This is especially true in the ever-changing world of PHP development.