Programming isn't just about writing lines of code; it's about crafting a functional, clean, and efficient masterpiece. Discover how simple hacks and smart practices can transform the coding journey from a simple task to an art form.
Stay tuned as we unravel tips that can help keep your code neat and reduce bugs, delve into debugging strategies, master version control, and identify time-saving shortcuts. We’ll also explore how leveraging libraries and frameworks can speed up development and discuss best practices to make your code future-proof.
Whether you're a beginner trying to find your footing or a seasoned developer looking for that extra edge, these tips and tricks are treasures waiting to be discovered.
- Simple Ways to Clean Your Code
- Debugging Like a Pro
- Mastering Version Control
- Time-Saving Shortcuts
- Leveraging Libraries and Frameworks
- Future-Proofing Your Code
Simple Ways to Clean Your Code
Writing clean code is an essential skill for any programmer. Whether you are just starting out or have years of experience, keeping your codebase tidy can save time and frustration down the line. Clean code is not just about following best practices but also about making your work easier to read, maintain, and scale.
First, always aim for clear and meaningful variable names. Instead of naming a variable ‘x,’ choose something more descriptive like ‘userAge’ or ‘totalAmount’. Descriptive names create self-documenting code, which helps others (and your future self) understand what the code does without needing additional comments. This approach aligns with insights from well-established programming experts.
Reducing the complexity of your functions is another effective way to keep your code clean. Functions should do only one thing and do it well. If you find a function doing too much, break it down into smaller, single-purpose functions. This increases readability and makes testing and debugging much easier. The goal is to have each function fit comfortably on one screen. This method is often emphasized by experienced coders.
“Clean code is simple and direct. Clean code reads like well-written prose. Clean code never obscures the designer's intent but rather is full of crisp abstractions and straightforward lines of control.” – Grady Booch, IBM Fellow
Consistency in coding style is crucial. Establishing and adhering to a standard coding style by using a style guide can help keep things uniform. Many languages and communities have their style guides, like PEP 8 for Python or the Google JavaScript Style Guide. Use automated tools like linters and formatters to enforce these styles to save time and ensure that your codebase remains consistent.
Comments can be your best friend or worst enemy. Useful comments explain why certain decisions were made, not just what a piece of code does. Avoid redundant comments, like ‘increment i by 1’ right next to ‘i++’, as they can clutter your code. Instead, focus on explaining the logic behind complex algorithms or business rules. This makes your comments more valuable and helps anyone reviewing your code understand the bigger picture.
Another principle for clean coding is to eliminate dead code. Unused variables, functions, and imported modules can bloat your codebase and make it harder to navigate. Regularly refactor and remove these unnecessary elements. This not only cleans up your code but also reduces the chances of bugs hiding in unused code paths.
Refactoring should be a regular part of your coding routine. Instead of letting the codebase degrade over time, set aside moments for cleanup. This might involve reorganizing classes, breaking apart large functions, or simply renaming variables for clarity. Remember, refactoring isn’t about changing functionality but about improving the structure.
If you follow these simple ways to clean your code, you’ll notice a significant improvement in your development process. Not only will your code be easier to understand and maintain, but it will also be more robust and scalable. These principles form the foundation of professional software development and are widely respected in the industry.
A small effort in maintaining clean code can lead to fewer bugs, happier teammates, and a smoother development experience. So grab your editor, and let’s make that code shine!
Mastering Version Control
Version control systems are the unsung heroes of software development, quietly keeping track of every modification to your codebase. They allow developers to work on features, fix bugs, and experiment with confidence, knowing that they can revert to a previous state if something goes wrong. One of the most widely used version control systems today is Git, which has become an industry standard. Understanding how to effectively use Git can save you time and headaches.
When managing version control, one of the most important concepts is branching. Branching allows you to create an independent line of development, so you can work on a specific feature or bug fix without affecting the main codebase. Once your work is complete and tested, you can merge it back into the main branch. It's a simple yet powerful mechanism to isolate and manage different streams of work.
Commit messages are also crucial. Every time you commit changes in Git, it's good practice to write a clear and concise commit message. This helps other developers (and your future self) understand the history of changes. A well-crafted commit message typically includes a summary of what was done and why. This can be incredibly helpful during code reviews and when tracking down bugs.
Another interesting aspect is the concept of 'pull requests' (or merge requests). This is a way of contributing your changes to a project. Before your changes are merged, they are reviewed and discussed by your peers. This collaborative approach not only ensures higher code quality but also spreads knowledge across the team. The review process can be a learning opportunity, offering new insights and perspectives.
“Version control is not just a tool, it’s a fundamental practice for any serious software development team.” - Linus Torvalds, creator of GitIncorporating continuous integration (CI) into your workflow can further enhance your use of version control. CI tools automatically test your changes and integrate them into the main codebase, ensuring that new commits do not introduce bugs. This automated testing can catch issues early, making your development process smoother and more reliable. Popular CI tools like Jenkins, Travis CI, and CircleCI can be easily integrated with GitHub or GitLab.
Advanced users might also explore 'rebasing,' another powerful feature in Git. Rebasing allows you to move or combine a sequence of commits to a new base commit. It's a great way to maintain a clean project history by eliminating unnecessary merge commits. However, it must be used cautiously, especially in shared branches, as it can potentially rewrite commit history.
To master version control truly, continuous learning and practice are essential. There are many resources available, including official documentation, tutorials, and community forums. Git itself provides a robust set of commands and functionalities, so becoming familiar with a comprehensive range of commands is recommended. By integrating these practices into your workflow, you'll find version control to be an indispensable tool that enhances productivity, collaboration, and code quality.
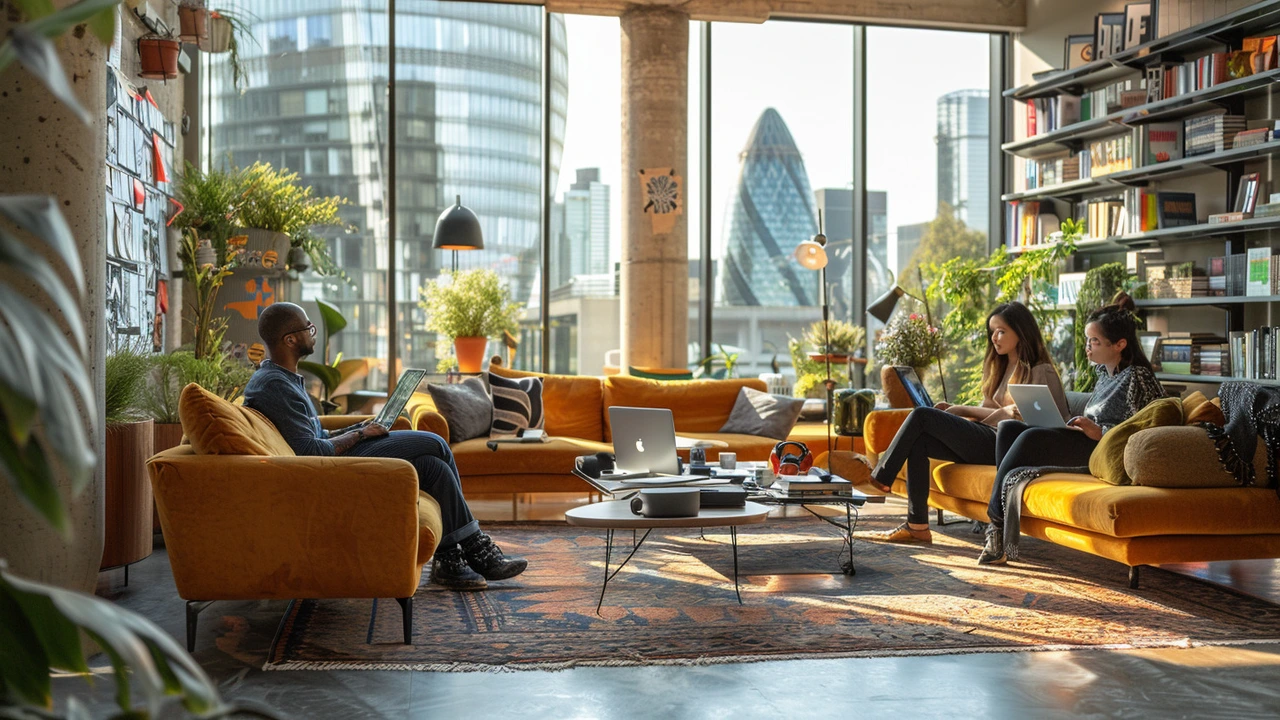
Time-Saving Shortcuts
Every programmer, from budding coder to seasoned developer, yearns for efficient ways to trim the extra minutes or even hours off of their workflows. Time-saving shortcuts are a key component in the toolkit of any effective programmer. These little tricks can drastically improve your coding experience by increasing your speed and productivity.
One of the best ways to save time is by mastering keyboard shortcuts in your Integrated Development Environment (IDE). IDEs like Visual Studio Code offer a wide range of shortcuts that can help you navigate through code faster. For instance, pressing Ctrl + P allows you to quickly open files by name. If you need to jump to a specific line, Ctrl + G comes in handy. These shortcuts, while small, can save you from repetitively navigating through menus.
"Efficiency is intelligent laziness." – David Dunham
Another aspect is the use of code snippets. Code snippets are prewritten pieces of code that you can insert into your own codebase. They can be especially useful for repetitive tasks such as creating function templates or boilerplate code. Custom snippets can be set up in most IDEs to fit your specific needs. This not only saves time but also helps in maintaining consistency across your projects.
Automation tools are also essential. Git hooks can automate tasks such as code linting, formatting, and running tests before commits. For example, using a pre-commit hook to run linters ensures that your code meets the team's coding standards before being committed.
Integrated tools like linters and formatters in your IDE are invaluable. They can automatically format your code according to predefined rules each time you save your file. This reduces the time spent on manual formatting and helps in keeping your code clean and readable.
If you often find yourself searching for some pieces of code you've written in other projects, consider using a snippet manager or a personal knowledge base. Tools like Dash or even a well-organized folder structure can save you a lot of time in finding that one perfect solution you know you've written before but can't quite place.
Another noteworthy mention is batch processing. Whether you need to run tests, deploy applications, or compile your code, doing it in bulk rather than one at a time can save substantial amounts of time. Build tools like Maven and Gradle, as well as deployment pipelines in Jenkins or GitLab CI, streamline multiple tasks making your development process more efficient.
Lastly, staying up-to-date with the latest shortcuts, plugins, and tools can give you an edge in efficiency. The programmer community is always evolving, and new tools are constantly being created to streamline workflows. Engage with the community to share and find fresh time-saving tips.
In essence, the magic of programming is often found in these small, seemingly trivial efficiencies that, when combined, can offer substantial time savings. Embrace these shortcuts and integrate them into your routine to enhance your productivity and enjoy a smoother coding experience.
Leveraging Libraries and Frameworks
Diving into the world of libraries and frameworks can seem daunting at first, but their benefits are undeniable. These tools are designed to speed up the development process by providing pre-written code that developers can use and integrate. Imagine working on a project without having to start from scratch every time; that's the power of leveraging these resources.
Consider React, one of the most popular JavaScript libraries out there. This tool allows developers to create dynamic and interactive user interfaces with ease. Instead of writing code to update the user interface manually whenever data changes, React takes care of that. The result is a smoother and more efficient coding process. Similarly, on the backend, frameworks like Express.js help streamline the creation of server-side applications by offering a robust set of features for web and mobile applications.
One fascinating fact is that many of the top tech companies heavily rely on these tools. For example, Facebook is rebuilt on React, and Netflix utilizes Node.js with Express.js for their server infrastructure. This shows their real-world applications and reliability. By using these libraries and frameworks, developers can focus more on solving specific problems and less on repetitive coding tasks.
"Frameworks are like your power tools, and libraries are the tools in your toolbox." – Peter Norvig
When choosing a library or framework, it's crucial to understand the problem you're trying to solve. Libraries offer more flexibility, allowing you to pick and choose functions that you need. On the other hand, frameworks provide a standard way to build and deploy your application, enforcing coding practices that might be beneficial for larger projects. For example, Django is a high-level Python framework that encourages rapid development and clean, pragmatic design.
It's also worth mentioning the **importance of regularly updating** your libraries and frameworks. Developers often release updates to fix bugs, enhance security, and improve performance. To ensure compatibility and to make use of the latest features, stay on top of these updates.
Moreover, it's astounding how extensive the documentation for these tools can be. Almost every well-known library or framework comes with comprehensive documentation and community support, which makes learning and troubleshooting much easier. For instance, the TensorFlow library for machine learning provides detailed guides, tutorials, and a vibrant community for support.
Incorporating these tools into your projects can also lead to increased productivity and code quality. Reusing well-tested and community-approved code allows you to avoid common pitfalls and focus on building unique features. Knowing when and how to leverage these can truly transform your development experience.
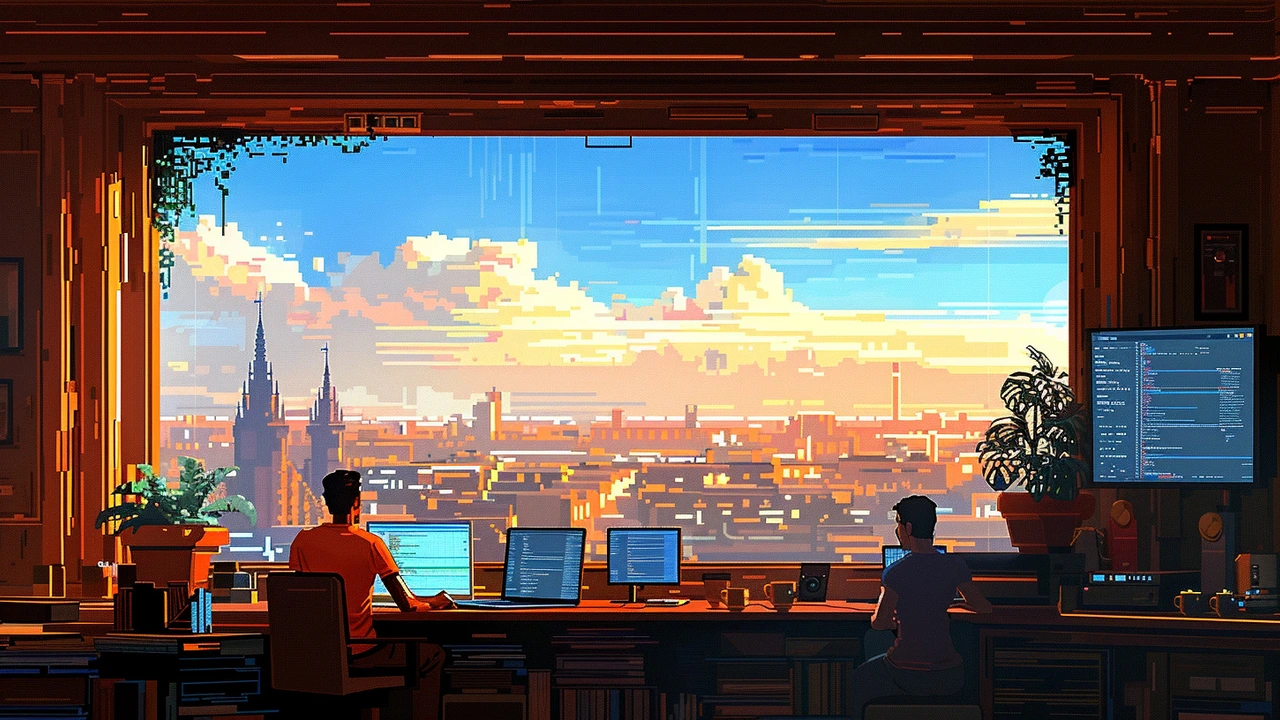
Future-Proofing Your Code
In today’s fast-paced technological world, ensuring your code stands the test of time is crucial. Future-proofing your code means writing it in a way that makes it adaptable and maintainable despite evolving technologies and requirements. The idea is to create code that's not only easy to read and modify but also enduring, reducing the likelihood of needing massive overhauls down the road.
One essential practice is to write clean and modular code. Breaking your code into small, manageable pieces makes it easier to understand and debug. Utilize functions and classes to encapsulate functionality. This separates concerns and makes your code more readable. For instance, if you are working on a large project, modularizing various parts into different files or modules can simplify maintenance and future enhancements.
Comments and documentation cannot be overstated. Despite being often overlooked, well-documented code can significantly aid in future-proofing. Documenting your reasoning behind complex logic and the purpose of different modules makes it easier for others (or yourself) to understand the code later. As per a study published in IEEE, around 40% of developers spend time understanding others' code. Proper documentation can save countless hours.
Another critical aspect is sticking to coding standards and best practices. Use consistent naming conventions for variables, functions, and classes. This consistency aids in readability and makes your code easier to navigate. Moreover, employing version control systems like Git can play a significant role in future-proofing. It not only keeps a history of changes but also allows multiple developers to collaborate without stepping on each other’s toes.
As Linus Torvalds, the creator of Linux, once said, “Given enough eyeballs, all bugs are shallow.”
The use of libraries and frameworks is often a double-edged sword. While they can significantly speed up development, be wary of becoming too dependent on a single library or framework that might become obsolete. Always prefer well-documented and widely-used libraries. Keep an eye on their updates and potential deprecation notices.
Automated testing is another future-proofing strategy. Writing tests for your code ensures that future changes won’t unintentionally break existing functionality. Use unit tests, integration tests, and end-to-end tests to cover different aspects of your application. Automated testing tools like Jest for JavaScript or JUnit for Java can be indispensable allies in maintaining the stability and reliability of your code.
Lastly, anticipate change. While it's impossible to predict every future requirement, writing flexible code that isn’t overly complicated or rigid can ease adaptation. Adopt principles like DRY (Don’t Repeat Yourself) and KISS (Keep It Simple, Stupid). These principles can help keep your codebase small and manageable, thereby more adaptable to change.
Remember, technology continually evolves, but with these practices, you can shield your code from becoming obsolete too quickly. Embrace these principles to ensure your hard work remains relevant and robust years down the line.