Python, a versatile and powerful programming language, has swiftly become a favorite among developers. Its simplicity and readability make it an excellent choice for anyone looking to dive into coding. This guide aims to arm you with essential Python tricks, tips, and techniques to help you navigate your coding journey.
Whether you're new to Python or looking to refine your existing skills, this article breaks down must-know tricks and more advanced features. We also touch on best practices to ensure you're writing clean and efficient code. Let's get started and make Python work for you!
- Getting Started with Python
- Must-Know Python Tricks
- Advanced Python Techniques
- Best Practices in Python Coding
Getting Started with Python
Python is not just a popular programming language; it's also one of the easiest to learn. With its clean and readable syntax, it has become a favorite for not only professionals but also for those who are just getting their feet wet in the world of coding. The language was created by Guido van Rossum and first released in 1991. Its design philosophy emphasizes code readability, and its syntax allows programmers to express concepts in fewer lines of code.
Before diving into coding, you need to install Python on your machine. Head to the official Python website and download the latest version. Python is available for all major operating systems including Windows, macOS, and Linux. The installation process is straightforward and the website offers detailed instructions for each system.
Once installed, you can write Python code using a simple text editor, but using an Integrated Development Environment (IDE) can make your coding experience smoother. PyCharm, VSCode, and Jupyter Notebook are some popular IDEs that offer features like syntax highlighting, code completion, and debugging tools. These can significantly speed up your development process.
"Python's ease of use and large community makes it the most recommended language for beginners." - Stack Overflow Developer Survey
One of the first steps in learning Python is understanding its basic data types and structures. Python has several built-in types like integers, floating-point numbers, strings, and booleans. Additionally, it offers complex data structures like lists, tuples, dictionaries, and sets. These structures allow you to store and manipulate data efficiently. For instance, lists are ordered collections of items, whereas dictionaries store data in key-value pairs.
Variables in Python do not need explicit declaration to reserve memory space. The declaration happens automatically when you assign a value to a variable. For example, if you write x = 5
, Python will create an integer object with the value of 5 and assign it to the variable x
. This dynamic typing makes Python very flexible but can sometimes lead to unexpected behaviors if you're not careful.
Running Your First Python Script
To get hands-on with Python, let's write a simple script. Open your text editor or IDE and type the following code:
print("Hello, World!")
Save the file with a .py
extension, for example, hello_world.py
. Open your terminal or command prompt, navigate to the directory where your script is saved, and run:
python hello_world.py
You should see the output: Hello, World!
. Congratulations, you've just written and executed your first Python script! This simple exercise demonstrates Python's simplicity and the immediate feedback you get from running your code.
As you continue your journey with Python, you'll discover its extensive standard library, which provides modules and functions for almost every task you can think of – from file I/O and system calls to web scraping and data visualization. Python's 'batteries-included' philosophy means you can get started quickly without needing to install many external libraries.
So, whether you aim to build web applications, automate tasks, or analyze data, Python's versatility and ease of use make it an excellent choice. Happy coding!
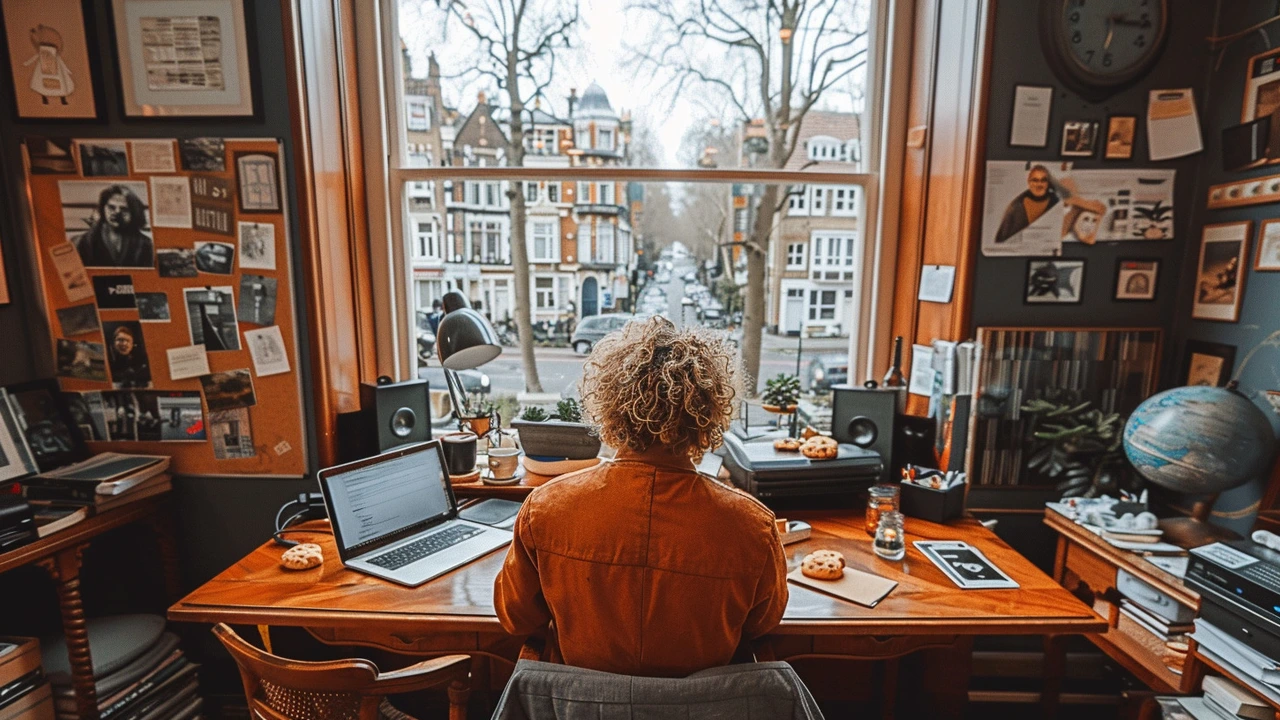
Must-Know Python Tricks
Learning Python can be incredibly rewarding, especially when you discover all the little tricks that can make your code cleaner, faster, and more efficient. One of the first things to understand is the use of list comprehensions. They provide a concise way to create lists. For example, instead of writing a for-loop to create a list of numbers, you can simply use [x for x in range(10)]
. This allows for more readable and compact code.
Another useful trick is the use of the enumerate() function. When you're iterating over a list and need the index of each item, enumerate()
comes in handy. It replaces the common pattern of using a separate variable to keep track of the index. For instance, for index, value in enumerate(my_list)
gives you both the index and the value directly in the loop.
Handling exceptions properly is another critical skill. Instead of just using a generic except
, you can catch specific exceptions to make your error handling more robust. For example, catching a ZeroDivisionError
separately from a ValueError
ensures that you handle different error conditions appropriately. This level of granularity makes your code more predictable and easier to debug.
"Learning Python is a dynamic and ongoing process; even seasoned programmers discover new tricks and techniques regularly." – Guido van Rossum
Using Dictionary Comprehensions
Much like list comprehensions, dictionary comprehensions can save you time and lines of code. Use them to create dictionaries in a straightforward way, such as {x: x**2 for x in range(10)}
, which creates a dictionary with numbers as keys and their squares as values. Simple and efficient!
Lambda Functions
Lambda functions are anonymous functions that you can define in a single line. While they shouldn't replace normal function definitions, they're helpful in situations where you need a small function for a short period. For instance, sorted(list_of_tuples, key=lambda x: x[1])
sorts a list of tuples by the second element, without needing a fully defined function.
A powerful feature of Python is its ability to handle complex data structures effortlessly. Nested lists and dictionaries can be created and manipulated with ease. Leveraging these capabilities can be a game-changer when dealing with large and complicated datasets.
If you are working with strings, don't miss out on the many built-in string methods. Functions like .strip()
, .lower()
, .join()
, and .split()
make string manipulation not only possible but very straightforward. These methods combined can simplify even the most complicated string handling tasks.
Finally, Python generators can massively streamline your code, reducing memory usage and keeping your programs efficient. Generators allow you to iterate over large datasets without loading them entirely into memory. This can be a game-changer in data-heavy applications. Use a generator expression by placing it within parentheses, like (x for x in range(10**6))
, which produces items one at a time as you iterate over them.
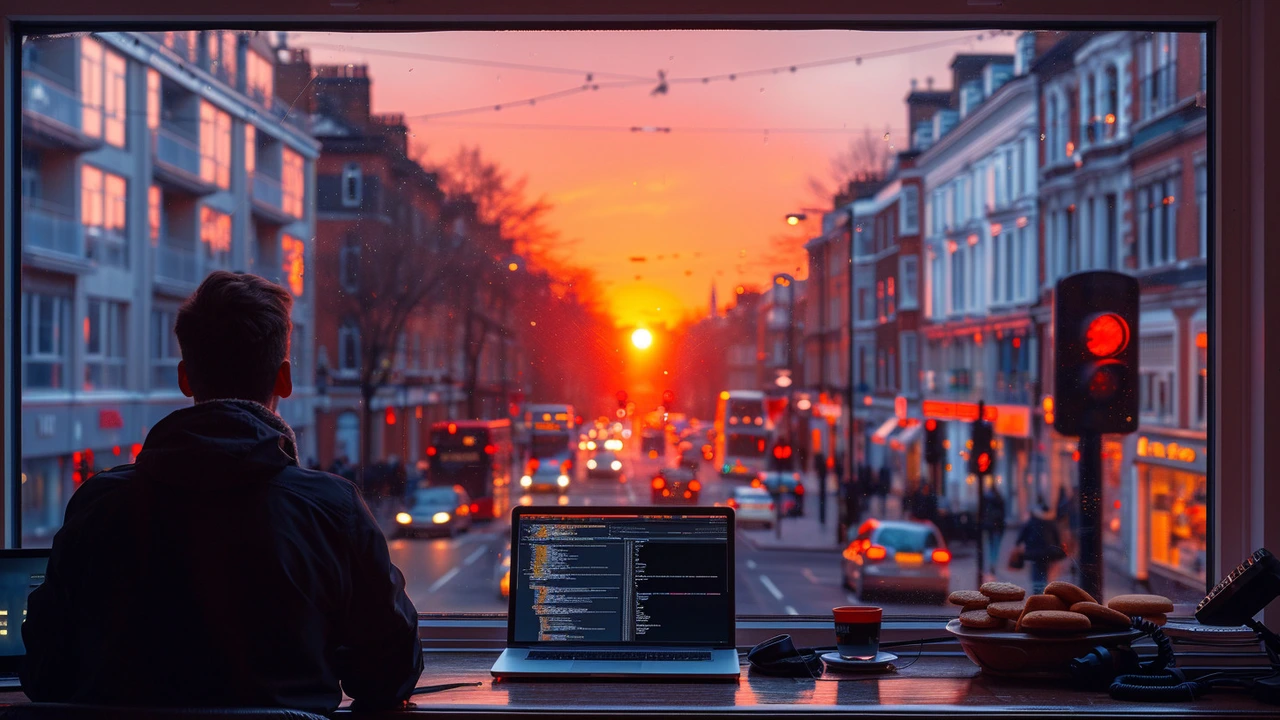
Advanced Python Techniques
Once you’ve grasped the basics of Python, it's time to dive deeper and explore advanced techniques that can make your coding more efficient and powerful. Understanding these techniques will not only enhance your skills but also open new doors to more complex projects and job opportunities.
1. List Comprehensions and Generator Expressions
List comprehensions provide a concise way to create lists. It’s a useful tool to convert lengthy code snippets into more readable and efficient code. For instance, imagine you need to generate a list of squares for numbers between 1 and 10. The usual approach might be to use a loop, but with list comprehensions, this process becomes streamlined: squares = [x**2 for x in range(1, 11)]
Generator expressions are akin to list comprehensions but with a fundamental difference: they use round brackets instead of square ones. This small change makes a huge impact in terms of memory usage, especially for large datasets, as generator expressions generate items one at a time rather than storing the entire list in memory. To get squares using a generator expression: squares_gen = (x**2 for x in range(1, 11))
2. Decorators: Improving Functionality
Decorators are a powerful feature of Python that allow you to modify the behavior of a function or method. They provide a simple syntax for wrapping and modifying functions. Here’s an example of a basic decorator that logs the execution time of a function: import time
def timer_decorator(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"Execution time: {end_time - start_time} seconds")
return result
return wrapper
Using the decorator: @timer_decorator
def some_function():
time.sleep(2)
print('Function execution')
When some_function()
is called, it will print the execution time, showcasing how decorators can be used to add functionality without altering the core logic of the function.
3. Context Managers and the 'with' Statement
When dealing with file operations and resources that need proper management, context managers are invaluable. They ensure that resources are properly cleaned up after use, even when errors occur. A common use case is opening and reading files. Instead of manually closing a file, you can use a context manager: with open('file.txt', 'r') as file:
data = file.read()
# file is automatically closed after this block
Creating custom context managers can further streamline resource management. A custom context manager is implemented using __enter__
and __exit__
methods or by using the contextlib
module:
According to Guido van Rossum, the creator of Python, context managers are one of the best tools for resource management, making code more readable and reliable.
Using contextlib
: from contextlib import contextmanager
@contextmanager
def managed_resource():
# Setup code
resource = acquire_resource()
try:
yield resource
finally:
# Teardown code
release_resource(resource)
4. Fun with Metaclasses
Metaclasses are a somewhat more advanced and esoteric feature of Python, but they can be incredibly powerful for certain tasks. Essentially, metaclasses allow you to control the creation of classes and customize their behavior. Below is an example of a simple metaclass that adds a 'greeting' method to any class it creates:
class GreetingMeta(type):
def __new__(cls, name, bases, dct):
dct['greeting'] = lambda self: "Hello from {}".format(self.__class__.__name__)
return super().__new__(cls, name, bases, dct)
Using the metaclass: class Person(metaclass=GreetingMeta):
pass
Now, calling person = Person()
will output 'Hello from Person'. Metaclasses are not something you'll use every day, but they can be a lifesaver for frameworks and libraries that need deep customization of class behavior.
print(person.greeting())
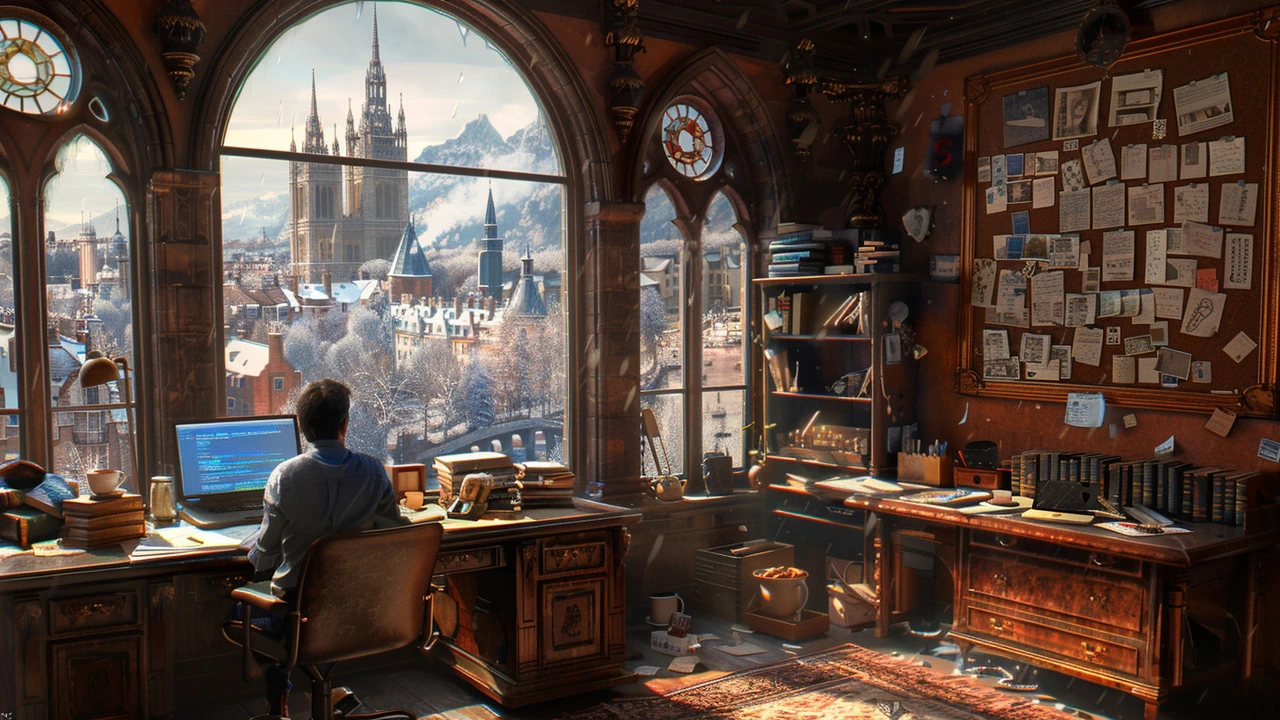
Best Practices in Python Coding
When it comes to *Python coding*, following best practices can make a world of difference. Not only does it help in writing more readable and maintainable code, but it also ensures that your programs run efficiently. Let’s dive into some actionable tips that can elevate your Python projects to a more professional level.
First, always adhere to the PEP 8 guidelines. PEP 8 is the style guide for Python code and it outlines how to format your Python scripts to maximize readability. Use four spaces per indentation level, and keep lines of code to a maximum of 79 characters. Consistency in style matters, and it's something that professionals take seriously.
Another essential practice is to write clear and concise comments. Comments are your saviors when you or someone else revisits the code after some time. Make sure your comments describe what the code is doing and, if necessary, why it is doing it that way. However, avoid over-commenting; let the code speak where it can.
Refactoring your code regularly also stands out as an important practice. By refactoring, you make the code easier to read and maintain without changing its behavior. Focus on removing code duplication, which not only simplifies the code but also reduces the chances of bugs. This often involves creating functions or classes, which brings us to our next point.
Make good use of functions and classes. If you find yourself writing the same code repeatedly, that’s a sign you need to create a function. The same applies to classes when dealing with complex data structures. Not only do functions and classes enhance code reusability, but they also make debugging easier.
Unit testing can’t be overlooked. Writing tests for your code ensures that it works as expected. Python’s built-in unittest module provides tools to test your code, and libraries like pytest can make testing even more comprehensive. Testing isn’t just a chore; it’s a powerful way to save time and headaches down the road.
Now, let’s talk about version control. Using tools like Git to track changes in your code is essential. Version control allows you to manage your code history, collaborate with others, and backtrack when issues arise. Never underestimate the power of having a reliable version control system in place.
Another best practice is to handle exceptions properly. Use try-except blocks to gracefully manage errors and avoid crashes. However, be specific about the exceptions you catch. Catching broad exceptions can hide bugs and make debugging more challenging. Explicit is better than implicit, as the Zen of Python suggests.
“Code is like humor. When you have to explain it, it’s bad.” — Cory House
Documentation is another cornerstone of valuable coding practices. Writing comprehensive documentation might seem daunting, but it’s incredibly useful. Document not only your code but also how to install and use your software. Tools like Sphinx can help automate and streamline the process of documentation.
Lastly, stay updated with the **latest Python features** and libraries. The Python community is vibrant and constantly evolving. Subscribe to Python newsletters, follow key contributors on social media, and participate in forums to keep your knowledge current. Embrace continuous learning as part of your coding journey.
By integrating these best practices into your routine, you can greatly improve the quality and efficiency of your Python code. Always aim for readability, maintainability, and reliability, and you'll find yourself coding like a pro in no time.