PHP, a popular scripting language in the world of web development, continues to evolve and adapt to modern programming needs. Whether you're crafting dynamic websites or developing complex applications, mastering PHP is essential for any web developer looking to create efficient, robust solutions.
In this article, explore a series of PHP tricks designed to enhance your coding efficiency. These strategies, gathered from years of coding experience, cover everything from optimizing your code's performance to utilizing PHP's built-in functions effectively. With these insights, you'll be able to streamline your workflow, reduce errors, and create more reliable applications.
By adopting these tips, developers can not only speed up their development process but also craft code that's easier to maintain. So, whether you're a seasoned expert or new to PHP, these techniques will offer something for everyone aiming to sharpen their coding skills.
- Understanding PHP Best Practices
- Optimizing PHP Code for Performance
- Leveraging PHP Built-in Functions
- Debugging Tips and Tools
- Working Efficiently with PHP Frameworks
Understanding PHP Best Practices
Diving into PHP development requires not just knowledge of the language's syntax but also a solid grasp of best practices. These practices are guidelines that help developers write clean, efficient, and secure code. One crucial aspect to consider is code readability. Writing code that others can understand easily is just as important as functionality. Clear and descriptive naming for variables, functions, and classes can make a world of difference. It's not just about making your life easier; it's about creating a community-friendly environment where code can be quickly passed on or picked up for improvements.
Another fundamental practice is adhering to coding standards like PSR-1 and PSR-2, which foster consistency across PHP projects. These standards set rules for code formatting, including spacing, indentation, and use of namespaces. Consistent formatting not only aids in readability but also minimizes the potential for errors. As we often need to collaborate with other developers, following such guidelines ensures everyone is on the same page, preventing friction and misunderstandings.
Security is another major concern when writing PHP applications. It's essential to regularly validate and sanitize inputs, especially when dealing with forms. SQL injection remains a significant threat, and using prepared statements is an effective way to shield against it. Also, never trust user inputs; always assume they could be harmful and code defensively. In this context, the principle of 'least privilege' should be applied when dealing with permissions: users and applications should have access only to the information and resources that are necessary for legitimate purposes.
According to Rasmus Lerdorf, the creator of PHP, "You don't necessarily have to write your entire application in PHP. The logic and features can be implemented in PHP, your high-load components can be in C.” This highlights the need for judicious use of PHP alongside other languages, especially when performance optimization is pivotal.
When it comes to optimizing your PHP code for performance, caching is your ally. Tools like OpCache can help significantly reduce the time it takes your scripts to run by storing precompiled script bytecode in memory, thus avoiding the need for PHP to load and parse scripts on each request. Caching frequently requested data, such as database results and API responses, can lead to a notable performance boost.
Moreover, utilizing exception handling properly can also contribute to maintaining robustness in PHP applications. Instead of allowing applications to fail unexpectedly, using try-catch blocks can help manage errors gracefully and provide clear error messages to users. This practice helps in debugging and offers a more professional user experience. Utilizing error logs systematically, rather than relying solely on error outputs, can further assist in uncovering issues before they escalate to end users.
Lastly, embrace version control systems such as Git, which are invaluable for managing and tracking code changes over time. This practice serves as a safeguard against unforeseen errors, allowing you to revert to earlier versions of your codebase whenever necessary. It also facilitates collaboration, enabling multiple developers to work on different aspects of a project without overwriting each other's work. Version control is not just a safety net; it's a proactive tool for managing the lifecycle of software development effectively.
Optimizing PHP Code for Performance
Coding in PHP allows developers to create dynamic and powerful web applications, but achieving peak coding efficiency requires deliberate optimization efforts. At the heart of performance optimization lies the understanding that every line of code contributes to the web application's responsiveness. Begin by streamlining your code: avoid redundancy and eliminate extraneous functions that might bog down performance. Each function or method should serve a specific purpose without reaching beyond its intended scope. This conscious simplification not only reduces processing times but also facilitates maintenance and debugging, ultimately refining the app's agility.
One effective method for enhancing PHP performance is through caching. Storing data temporarily using caching techniques can minimize expensive database calls and accelerate data retrieval. PHP offers several caching options, including opcode caches like OPcache, which caches precompiled script bytecode, leading to faster execution times. By leveraging these tools, developers can dramatically cut down the time taken to process requests, enhancing user experiences with quick loading pages.
"The key to optimizing PHP scripts is not just in the code itself, but in understanding how that code interacts with the server and the database," says Rasmus Lerdorf, the creator of PHP.
Tuning the configuration of your PHP environment is also essential. Ensure that your PHP version is up to date, as each release typically includes enhancements that could boost performance. PHP 8, for instance, introduced the JIT (Just-In-Time) compiler, which translates code on the fly into a lower-level machine representation, significantly speeding up executions. Pay attention to your memory limits and maximum execution time settings—these elements can greatly affect how efficiently your applications perform under load. Adjust them as necessary based on the nature of your application and the expected traffic.
Database Interactions
Interfacing with databases often constitutes a significant portion of a PHP application's workload. Optimize these interactions by using prepared statements for database queries, thereby improving security and reducing query parsing time. Where possible, choose indexed data retrieval, as indexes can substantially boost data retrieval speed. If you're dealing with large datasets, consider implementing pagination to avoid overwhelming server resources and reducing load times for users. These strategies, when systematically applied, can create a more responsive application that scales gracefully under increased demand.
Monitoring and profiling your PHP applications can reveal latent areas for improvement. Tools like Xdebug and Blackfire provide insights into processing bottlenecks and execution paths, allowing you to identify and rectify inefficiencies. Regular performance audits armed with these insights ensure your applications remain nimble and competitive. Groom your codebase with iteration, reducing the footprint where possible and rendering your PHP tricks more potent and effective.
Using Built-in Functions
PHP's arsenal of built-in functions can be another ally in optimizing your code. By employing functions that are natively optimized, such as array functions, string manipulation functions, and mathematical operations, you can achieve efficiencies not possible with handwritten loops or constructs. These functions, written in C within the PHP core, often do the heavy lifting in less time and with fewer resources than custom solutions. Remember, PHP development doesn't just stop at writing working code—enhancing the code's performance bridges the gap between functioning and flourishing applications.
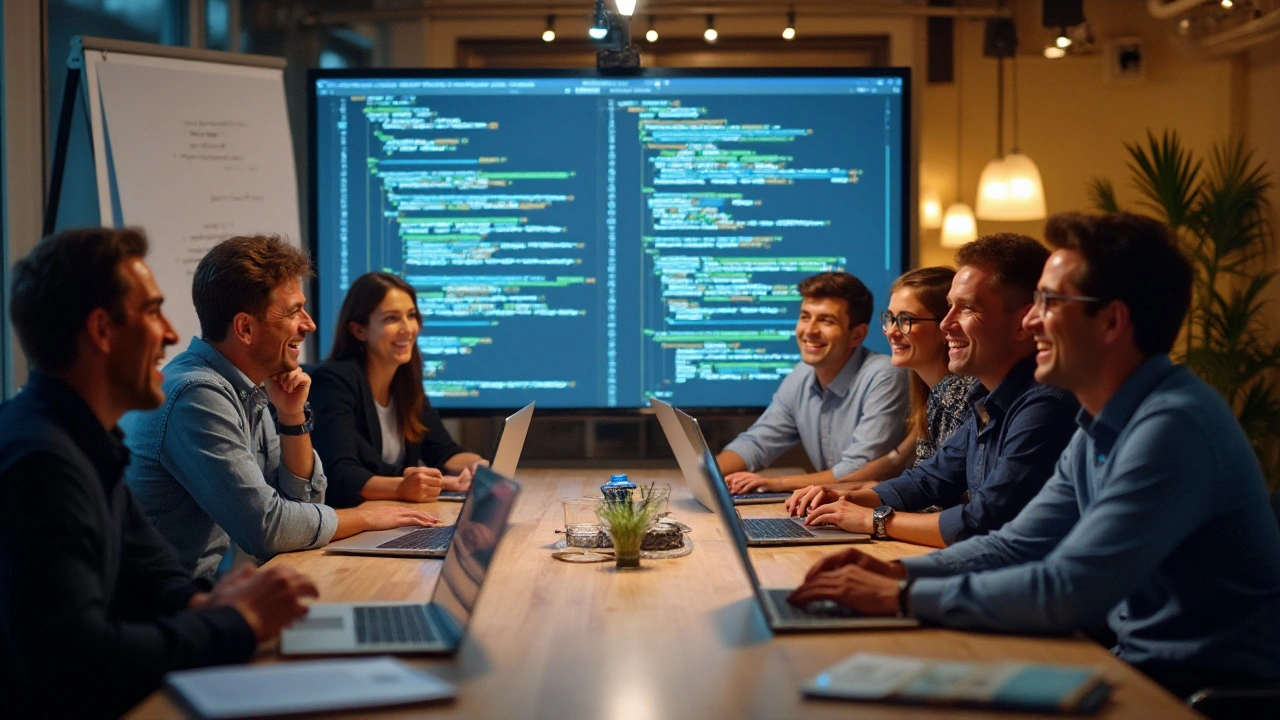
Leveraging PHP Built-in Functions
In the bustling world of PHP development, utilizing PHP built-in functions can be a real game-changer for developers aiming to enhance their coding efficiency. These functions are pre-defined and offer a plethora of options to handle data, manage arrays, interact with files, and much more. They are like the pre-packaged power tools in a developer's toolkit, allowing you to achieve tasks without writing code from scratch.
Packing a punch with their versatility, PHP's built-in functions like `implode()`, `explode()`, and `str_replace()` offer powerful mechanisms for string manipulation. For instance, you can convert an array into a string or vice-versa with `implode()` and `explode()`, respectively. This magic of conversion not only saves time but also minimizes the risk of errors that can occur during manual conversions. Such conversions are pivotal, especially when dealing with database operations or parsing user data from forms.
"Efficiency is doing things right; effectiveness is doing the right things." - Peter Drucker
Working with array data is another area where PHP built-in functions shine through. Functions like `array_filter()` and `array_map()` provide developers the ability to elegantly manipulate arrays. Instead of writing loops to sort or filter through arrays, these functions accomplish the same with a single line of code, improving the readability and maintainability of your scripts. These functions are not just about saving code lines; they enable developers to work smart, navigating through complex data structures efficiently.
Exploring Mathematical Functions
PHP does not fall short with mathematical functions either, offering a suite of tools for tasks ranging from simple calculations to more complex mathematical operations. Functions like `round()`, `ceil()`, and `floor()` help in managing decimal points effectively, while others like `abs()` and `sqrt()` handle absolute values and square roots, respectively. These can be engineered into applications requiring precise mathematical operations, such as financial software or scientific applications.
Taking a step further, PHP’s ability to handle mathematical functions can extend into statistical operations as well. Imagine running a website with various product ratings, and you're tasked with calculating an average rating from large datasets consistently. The coding efficiency you gain from PHP's mathematical arsenal allows you to perform these operations seamlessly, ensuring not only accuracy but also speed.
Utilizing Date and Time Functions
Date and time management is a fundamental aspect of many applications, and PHP provides comprehensive functions to undertake this task effectively. Functions like `date()`, `strtotime()`, and `mktime()` enable developers to handle and manipulate time-related data with ease. For example, converting human-readable dates into timestamps can be effortlessly done using `strtotime()`, which is indispensable for storing dates in databases or comparing two dates.
PHP’s built-in date and time functions allow developers to perform tasks of varying complexity, be it generating time stamps for logging actions within an application, calculating the difference between two dates, or even formatting dates into different styles. These functions not only simplify how dates and times are handled but also ensure you do not get bogged down by different formats or regional settings.
The secrets to harnessing PHP's built-in functions lie in understanding their potential and integrating them into your development process. By leveraging the function library PHP offers, you make your scripts more efficient, robust, and reliable, which in turn boosts your productivity and lets you focus on developing features that matter the most. The real magic happens when you employ these functions to unlock new possibilities and creative solutions within your PHP projects.
Debugging Tips and Tools
In the realm of PHP programming, debugging is a crucial skill that can save both time and sanity. Anyone who has spent late nights chasing down a pesky bug knows the importance of having a reliable strategy and the right tools. Debugging effectively requires a combination of technical know-how, patience, and precise tools tailored to work seamlessly with PHP.
First, embracing a debugging mindset grounded in systematic thinking can be incredibly valuable. Recognizing patterns, isolating the problem by breaking down code into smaller, manageable parts, and testing each component individually is a fundamental approach. A common starting point is to thoroughly review each line of code, verifying logic, syntax, and flow. Keeping accurate documentation about changes made during this process can prevent revisiting the same mistakes.
One of the most direct tools at a developer's disposal is PHP's native error reporting capabilities. Activating error reporting is akin to switching on a flashlight in a dark tunnel. By editing the php.ini file or incorporating php commands like ini_set()
and error_reporting()
, developers can catch errors early on. Display errors can be enabled to output error messages directly during development. However, exercising caution is crucial as exposing errors can potentially reveal sensitive information in a live environment.
"The sooner you start coding away, the longer the program will take." – Roy Carlson
Beyond native error tools, integrating sophisticated debugging tools such as Xdebug can exponentially enhance productivity. Xdebug provides advanced insights including stack traces, breakpoints, and local variable inspection. It supports remote debugging, allowing developers to inspect the running application from their preferred Integrated Development Environment (IDE). Configuration of Xdebug can be complex, but detailed guides and community forums are rich resources for any difficulties encountered.
Furthermore, employing logging as a routine practice can preemptively catch issues before they escalate into bugs. PHP’s error_log()
function allows error messages to be directed to a file, providing a historical reference for debugging. Coupling this practice with log monitoring systems can alert developers to anomalies in real-time, reducing the time between bug discovery and resolution.
Developers might also consider using third-party applications such as Laravel Debugbar for framework-specific projects. Such tools provide a real-time look at queries, routes, and performance metrics right in the browser, offering a consolidated view of the intricacies within the project. Understanding how these requests interact with caching and session data can provide insights that are not immediately apparent from the code.
Let’s not forget the community aspect – turning towards forums and other online platforms like Stack Overflow can unveil solutions previously unconsidered. In these spaces, developers exchange insights freely, providing a network of support that's particularly useful when new challenges arise. It's as much about learning from other people's past experiences as it is about solving immediate problems.
Tool | Description | Use Case |
---|---|---|
Xdebug | An advanced debugger extending PHP capabilities | Deep, interactive debugging and profiling of code |
PHP Error Log | Native logging capabilities to track errors | Error tracking and historical bug analysis |
Laravel Debugbar | A package for Laravel projects showing debugging metrics | Real-time query and session data examination |
Ultimately, mastering debugging in PHP is as much about knowing the right tools as it is about cultivating a problem-solving attitude. The ability to detect and correct bugs effectively often distinguishes an average developer from an exceptional one in the fast-paced world of web development.
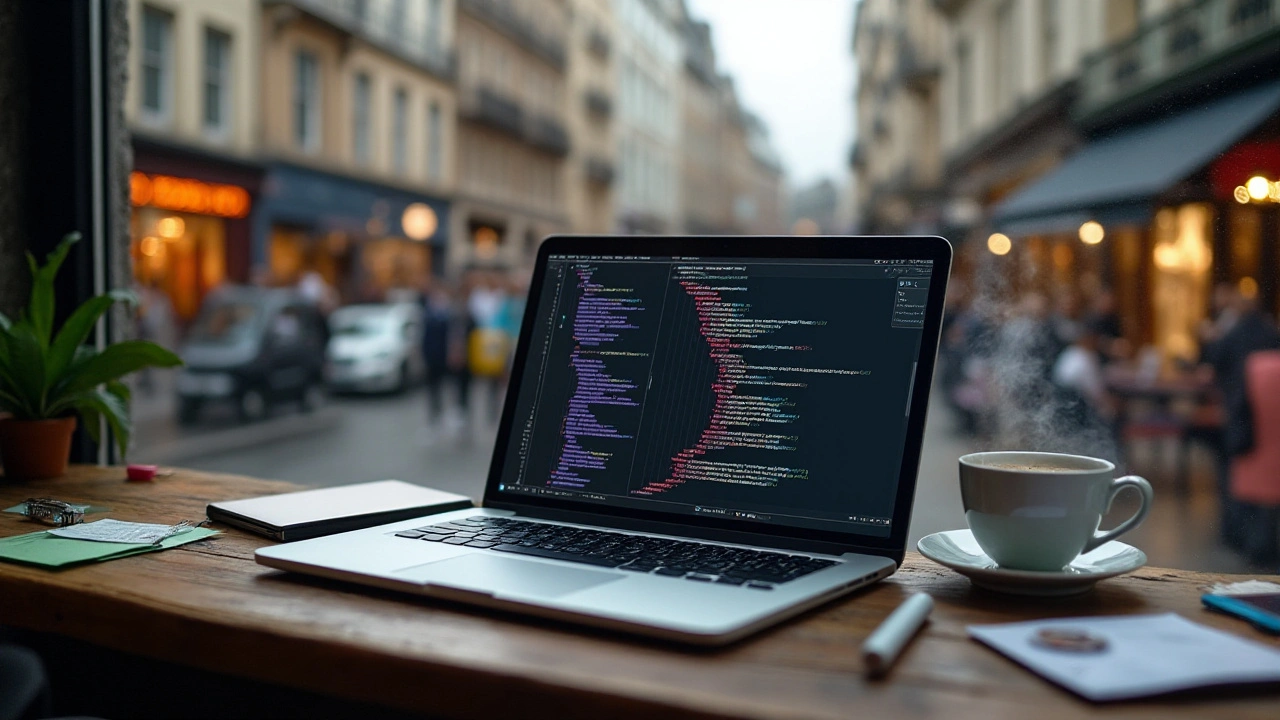
Working Efficiently with PHP Frameworks
In the ever-evolving landscape of web development, leveraging PHP frameworks can significantly enhance your coding efficiency and accelerate project delivery. PHP frameworks, with their ready-to-use libraries and reusable components, provide a structured programming foundation that allows developers to focus on building applications rather than solving repetitive code challenges. This approach not only saves time but also fosters best practices, ensuring the codebase remains organized and efficient.
Perhaps the most popular PHP framework, Laravel, offers expressive, elegant syntax that has revolutionized the way we think about PHP development. Its powerful features, such as Eloquent ORM, Blade templating engine, and built-in unit testing, simplify complex tasks and improve the workflow of any PHP project. PHP tips often emphasize the importance of using such frameworks, as they can substantially reduce the amount of boilerplate code, making applications more maintainable and scalable.
"The beauty of using a framework lies in its ability to bring consistency and reliability to your development process," notes Taylor Otwell, the creator of Laravel. "By following established conventions and utilizing the rich ecosystem of packages, developers can avoid pitfalls typically encountered when building applications from scratch."
Frameworks like Symfony and CodeIgniter also deserve mention, each catering to different needs and preferences. Symfony, known for its extensive documentation and best-in-class components, excels in enterprise-level applications, while CodeIgniter offers a lightweight, easy-to-learn platform ideal for simple applications. The key to maximizing these tools lies in understanding their strengths and applying them judiciously to your projects. This requires an initial learning curve, but the long-term benefits in terms of efficiency and professionalism are well worth the effort.
Working with PHP frameworks involves understanding their architecture and conventions. MVC (Model-View-Controller) is a standard pattern employed by these frameworks, aiding in separating business logic from the user interface, thereby enhancing code readability and maintainability. Developers are encouraged to explore the best practices recommended by these frameworks and integrate them into their coding habits. There's also a growing trend towards full-stack frameworks like Symfony, which offer integrated solutions covering everything from templating to dependency management, helping teams work cohesively and efficiently.
To illustrate the impact of using frameworks on project timelines, consider the initial setup phase. With frameworks, scaffolding a new project takes minutes instead of days, thanks to their command-line tools and pre-configured settings. This allows developers to hit the ground running, concentrating on delivering features that drive business value. Additionally, leveraging community-contributed packages or modules can enhance functionality without having to reinvent the wheel.
Framework | Primary Benefit | Popularity |
---|---|---|
Laravel | Comprehensive tooling, robust ecosystem | High |
Symfony | Reusability, enterprise-level applications | Medium |
CodeIgniter | Simpler, minimal footprint | Medium |
In conclusion, to maximize your coding efficiency with PHP frameworks, delve into their core features, engage with their active communities for updates and support, and continually refine your skills in their usage. By doing so, you'll not only enhance your productivity but also ensure that your web applications are built on a solid, reliable foundation. The journey might seem intricate, but the reward—being a more efficient and successful PHP developer—is a worthy pursuit.