Ever wondered how some people seem to breeze through Python coding while you’re stuck pondering over a loop? The secret often lies in the little tricks and tips that can transform your coding practice from average to amazing. We’re diving into some nifty Python tricks that could do wonders for your programming journey.
First up, list comprehensions. A real game-changer, list comprehensions make your codes not only more readable but also more efficient. Instead of writing those overwhelming nested loops, you can get what you need in just a line or two. It’s all about getting the job done while keeping things neat and tidy.
And then there are lambda functions. I know, the name might sound intimidating, but they can simplify your life significantly. These are anonymous functions you can whip up quickly without the formalities of a regular function. They're perfect for those moments when you need a small, throwaway function.
So, if you’re ready to elevate your Python skills and explore how these tips can make your coding life a breeze, stick around. Because once these tricks are under your belt, there’s no stopping what you can achieve in Python!
List Comprehensions
Ever feel like your code gets bogged down by loops and more loops? That's where list comprehensions come in handy. They're your go-to trick for making Python code more concise and readable. Instead of the old-school way of writing lengthy loops to transform or filter lists, list comprehensions let you do it all in one line. Talk about efficiency!
Here's how it works. Let's say you have a list of numbers and you want to create a new list with each number squared. The traditional way would mean setting up a loop, initializing an empty list, and then appending squared numbers one by one. But with list comprehensions, it's as simple as:
numbers = [1, 2, 3, 4, 5] # Your list of numbers
squared = [x ** 2 for x in numbers] # Voilà!
And there you have it, your squared list with minimal fuss. But it doesn't stop there. You can add conditions into the mix too. Imagine wanting only the even numbers from your list squared. Easy-peasy with addition of an if condition:
even_squared = [x ** 2 for x in numbers if x % 2 == 0]
By doing this, you've filtered through the list, grabbing only even numbers before squaring them. List comprehensions are all about making your code shorter, faster, and way cleaner.
Advantages of List Comprehensions
- Readable and Concise: They pack a lot of punch in a single line, making your code easier to understand at a glance.
- Performance Boost: Generally, list comprehensions run slightly faster than traditional loops because they’re optimized for the task.
- Flexibility: You can manipulate data, apply transformations, and filter lists effortlessly.
It’s like having a Swiss Army knife for handling lists in Python. Once you start using them, there's no turning back. They bring simplicity and elegance, which are key ingredients to mastering Python.
Lambda Functions
Alright, let’s talk about Python lambda functions. These are often a favorite tool among programmers for their simplicity and effectiveness. Think of them as super-efficient mini-functions that you can create on the fly. You won’t believe how handy they are until you start using them.
A lambda function is a small anonymous function, consisting of a single expression. Here’s what makes them special: they can take any number of arguments, but can only have one expression. Sounds a bit restrictive? Well, that’s their power—they’re incredibly concise and designed for short operations.
Why Use Lambda Functions?
Lambdas are all about efficiency and speed, perfect for situations where you need a quick, one-off function without going through the whole process of defining a function with the def
keyword. This makes them ideal for simple operations that are done repeatedly.
A common use for lambda functions is in data processing with functions like map()
, filter()
, and reduce()
. They allow you to execute fast, single-threaded operations on data streams, making your coding even more efficient.
Writing a Lambda Function
Here’s how you can whip up a quick lambda function:
add = lambda x, y: x + y
In this example, add
is a lambda function that takes two arguments x
and y
and returns their sum. Super neat, right?
Real-World Example
Let’s see an example with the filter()
function. Imagine you have a list of numbers, and you want to filter out the even numbers:
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
So here, the lambda function is quickly identifying and filtering the even numbers from the list. It’s clean, simple, and powerful.
When Not to Use Lambdas
While they’re efficient, lambda functions aren’t always the right choice. If your function is complex or will be used repeatedly across your application, it's typically better to write a standard function with the def
keyword. Remember, lambdas are great for simple expressions, but for anything beyond that, consider sticking to traditional functions.
Give lambda functions a try the next time you're looking to accomplish something quick and straightforward. Integrating them into your development practice can be a big win for your coding efficiency.
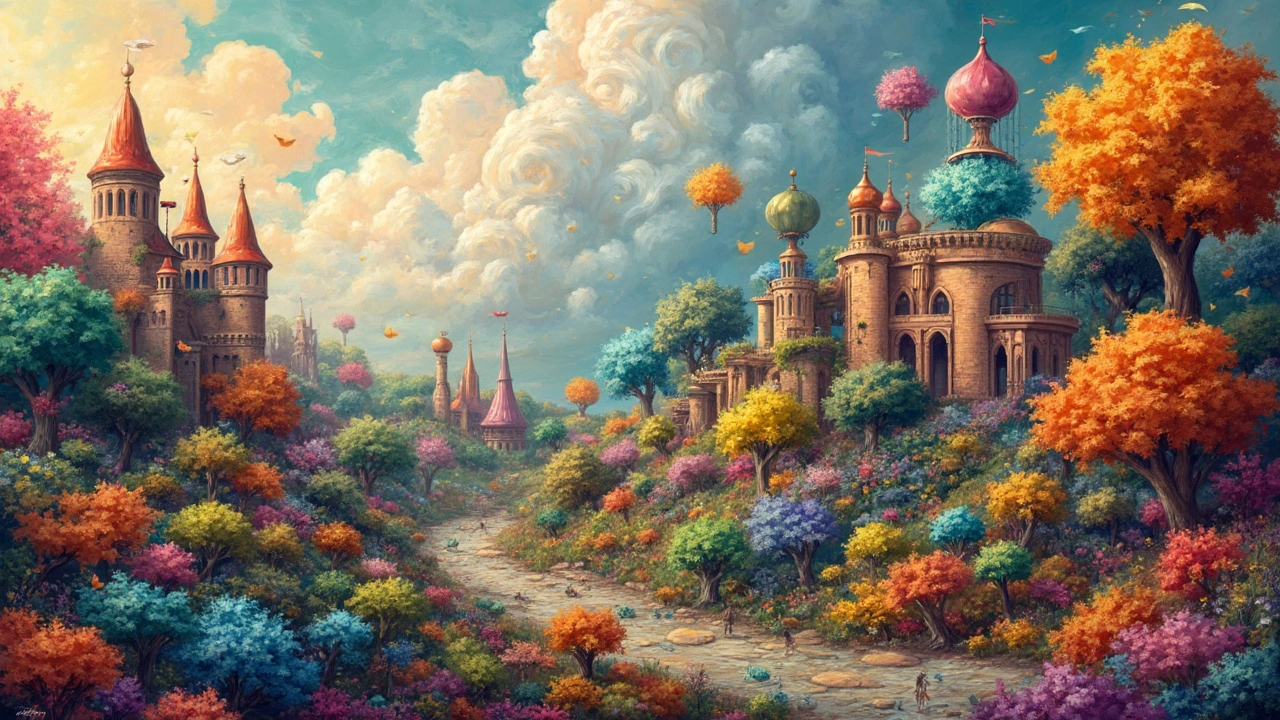
Magic of Python Libraries
Python is downright famous for its extensive libraries, and for good reason. If you've ever marveled at how someone can solve complex puzzles in a snap, it might just be the work of the right library. They're like cheat sheets that help you skip some heavy-lifting and get straight to the fun stuff of development.
Let's dive into some of the coolest Python libraries out there. One name you can't ignore is NumPy, especially if you're into data science. It lets you perform complex mathematical operations with ease, and if you're dealing with arrays, it's a lifesaver.
Then there's Pandas. It's all about data manipulation and analysis. You can handle big data sets and make nifty filters and data views. It feels like having a superpower when working with tons of data without losing your mind.
Visualization Libraries
On the more visual side of things, Matplotlib and Seaborn come to the rescue for all things plotting. Matplotlib is the classic choice for creating static, interactive, and animated visualizations, while Seaborn takes it a notch higher with some amazing themes and sleek graph styles.
If you're working on web applications, Flask and Django offer some of the best frameworks to make your life simpler. Flask is perfect for small projects and quick prototypes due to its lightweight nature. On the flip side, Django is like a full-on web powerhouse, providing built-in authentication, routing, and database manipulation.
Library | Use Case |
---|---|
NumPy | Mathematical operations |
Pandas | Data analysis |
Matplotlib | Visualization |
Flask | Web applications |
Harnessing the power of Python libraries can streamline coding and allow you to focus more on application logic and less on redundant coding tasks. Libraries save developers hours and sometimes even days of coding. They're not just important—they're essential.
Error Handling Tips
Diving into error handling in Python? Trust me, it's one of those things that can save you tons of time (and headaches). When you think about coding, errors are like those unavoidable roadblocks. But here's the good news—Python gives you awesome tools to manage these hiccups.
Understanding Python Exceptions
First off, let’s decode Python exceptions. Imagine exceptions as flags that tell you something’s off. A common exception you might encounter is a 'ZeroDivisionError', for instance when someone accidentally tries dividing by zero. Python kindly throws these flags at you with a helpful description so you know what went wrong.
Using Try-Except Blocks
One cool trick: the try-except block. It’s like a safety net for your code that catches issues before they escalate. Here’s how to make use of it:
- Try Block: Wrap the code that might cause an exception.
- Except Block: Decide what should happen if an exception occurs. Redirect the error or log it responsibly.
For instance:
try:
result = 1 / 0
except ZeroDivisionError:
print("Hold up, you can’t divide by zero!")
In this snippet, Python dips into the try block, realizes there’s a problem, and neatly takes you to the except block without crashing your program.
Finally: The Cleanup Crew
Don’t forget the “finally” clause. This part of your code runs no matter what—whether you’ve fixed the error or not. It's perfect for cleanup tasks like closing files or releasing resources.
Don't Ignore Logging
Pro tip: use logging. Logs are like breadcrumbs you leave behind to retrace your steps. The logging module in Python lets you document errors efficiently, helping you hunt down and fix bugs faster.
Here’s a quick look at how you could use it:
import logging
logging.basicConfig(level=logging.DEBUG)
try:
x = some_undefined_variable
except NameError as e:
logging.error("Got a NameError: %s", e)
With Python's built-in tools, handling errors becomes more of a smooth ride than a bumpy road. Remember, practice makes perfect. The more you incorporate these tips, the more Python becomes your friend rather than a mystery.