Ever thought about how some programmers just breeze through their code like they were born to type? Well, chances are, they’re not magic—they just know some sweet programming tricks. Getting the right tricks under your belt can change the way you code, making you quicker and more efficient. From nifty keyboard shortcuts to smart debugging strategies, it’s all about making life a little easier.
Keyboard shortcuts, my friend. They’re a coder’s best ally, shaving precious seconds off those repetitive tasks. Imagine copying, pasting, or finding code in the blink of an eye. Start with the basics and build your way up—before you know it, you'll be slicing through your workflow like a hot knife through butter.
- Quick Keyboard Shortcuts
- Code Optimization Hacks
- Understanding Algorithms
- Debugging Tips
- Keeping Up with Trends
Quick Keyboard Shortcuts
You know, one of the fastest ways to level up your coding game is to get comfy with keyboard shortcuts. Seriously, these little combos can save you from drowning in a sea of clicks and menus. Once you start using them, you’ll wonder how you ever coded without them.
Let’s say you’re working in a popular code editor like VS Code. Here are some superstar shortcuts that’ll really speed things up:
- Ctrl + C and Ctrl + V: Copy and paste are your bread and butter. Use Ctrl + Shift + V to paste without reformatting.
- Ctrl + D: Got a recurring variable name? Highlight it once and hit Ctrl + D to select the next occurrence. Boom, edit them all at once.
- Ctrl + /: Need to comment out a block of code quickly? This shortcut will toggle comments on the selected line(s).
- Shift + Alt + Down Arrow: Want to duplicate a line of code? This combo will copy it right to the line below.
For macOS users, swap out Ctrl with Command for most of these shortcuts. You’ll get the hang of it in no time.
Here’s a quick look at how much time you can save:
Task | Clicks with Mouse | Keystrokes |
---|---|---|
Copy/Paste Text | 3 | 1 |
Duplicate Line | 4 | 2 |
Comment Code | 5 | 2 |
That’s a lot of saved time, right? Start with a few shortcuts and gradually add more. Your fingers will start moving faster than your brain before you know it.
Code Optimization Hacks
When it comes to coding, less is often more. That's why code optimization hacks are crucial for making your software snappier and more efficient. Let's talk about some practical ways to trim the fat and make your code run smoother.
First up, consider simplifying your algorithms. Sometimes, the simplest solution is staring you in the face. Instead of over-engineering, break down the problem and see if there’s a straightforward algorithm that fits better. Remember, the goal is to save on processing time and memory without sacrificing functionality.
Next, let's dive into the world of loops. Avoid those nested loops if you can. They can be real performance killers. Instead, look for ways to flatten them out. Use data structures like hash maps, which can help you reduce time complexity.
Minimizing the use of global variables is another trick. They might seem convenient, but they can slow down your code execution and are hard to track. Opt for local variables whenever possible, and you’ll notice a bump in speed.
- Use compiler settings that optimize your code. Most modern compilers offer flags for optimization. Do a bit of research on your specific compiler to enable these settings.
- Profile your code regularly. Tools like gprof can help you identify bottlenecks where your application spends the most time. It’s about working smart, not hard.
- Cache results of expensive operations if they're used repeatedly. By storing these results, you'll save time and reduce needless computation.
Consider this: a study showed that compressing images and using smaller data types could shave off significant load times in web applications. While software development isn't just about shaving milliseconds off everything, sometimes these tiny tweaks add up to a huge performance boost.
Wrapping it all up, remember that optimizing code is an ongoing process. Keep refining and looking for more coding hacks that fit your needs. It's all about making your code work harder, so you don’t have to.
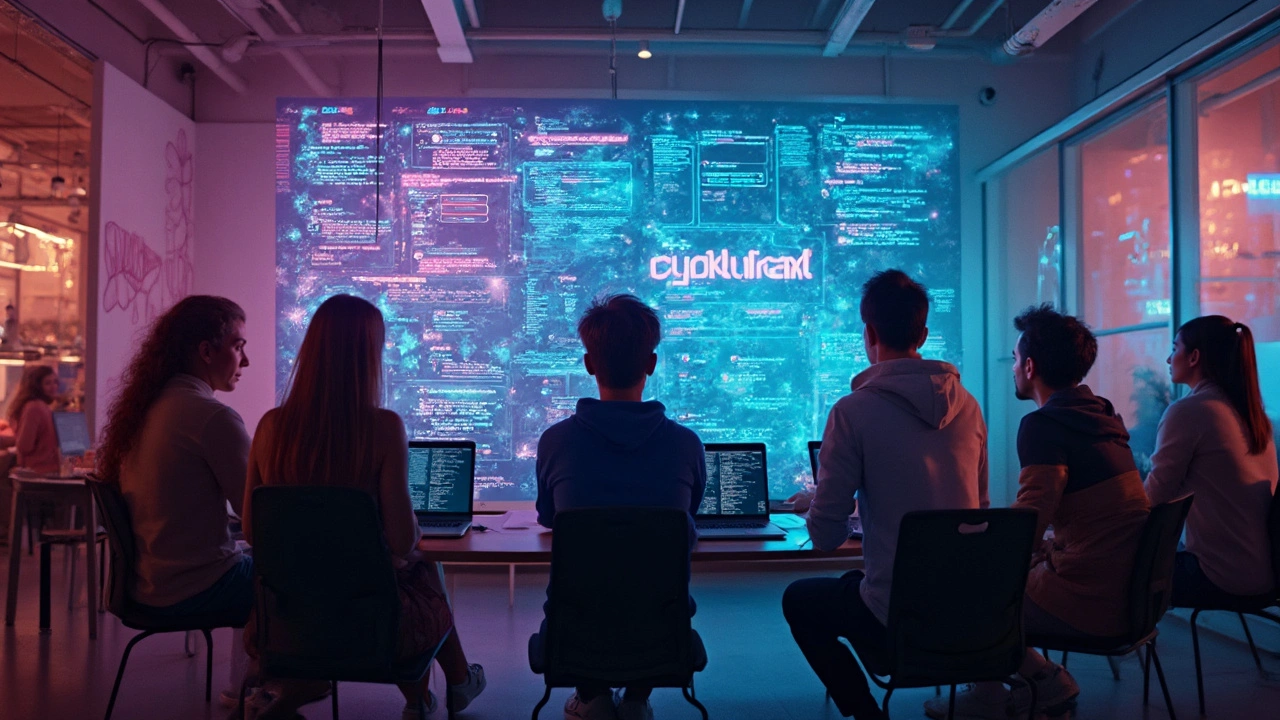
Understanding Algorithms
Alright, algorithms. They're kind of the backbone of coding and figuring them out can seriously up your game. An algorithm, simply put, is a set of instructions to solve a problem or accomplish a task. It's like a recipe for your program, laying out exactly what steps to take and in what order.
So, why sweat over algorithms? Well, they’re at the core of software development. Whether you’re sorting data, searching for an item, or just trying to calculate something efficiently, a solid understanding of algorithms will get you there faster and more efficiently.
Let’s chat about a couple of basic ones:
- Bubble Sort: This one’s like the training wheels of sorting algorithms. It compares elements side by side and swaps them if they’re in the wrong order. Though not the fastest, it’s super easy to get your head around.
- Binary Search: If you’re searching through a sorted list, binary search is your best bet. It cuts the problem size in half each step of the way, making it incredibly fast compared to linear searching.
Now, both of these are just the tip of the iceberg. To really grab hold of what makes algorithms tick, you gotta think about time complexity. This helps you understand how the speed of an algorithm changes with input size. For instance, Bubble Sort generally has a time complexity of O(n²), while Binary Search is O(log n), meaning it scales way better with larger datasets.
To wrap it up, if you’re diving into coding and want to solve problems speedily and smartly, getting cozy with algorithms is definitely worth your time. They're essential for debugging, optimizing code, and even during those tricky coding interviews. So, go on, roll up your sleeves and start exploring these coding hacks and tricks!
Debugging Tips
Debugging is like solving a mystery—only you’re the detective, and the clues are hidden in your code. Ever spent hours trying to figure out why your program’s acting up? You’re not alone. Let's dive into some handy tricks to make those bugs less of a headache.
First things first, understand the bug. Sounds simple, right? But this is where most people trip up. Don’t just rush to fix it—take time to analyze what's going wrong. Read error messages closely; they’re actually quite helpful if you pay attention. They often point you right to the culprit.
Isolate the issue by breaking down your code into smaller segments. Think of this as a “divide and conquer” strategy. Run each section separately if needed. This helps pinpoint which part is misbehaving without the distraction of the whole system.
Now, let’s talk tools. Your IDE (Integrated Development Environment) is your best friend here. Ever tried using breakpoints? They let you pause code execution and examine the state of your application at specific moments. Handy, right? Most popular IDEs have built-in debuggers designed to help you track down issues fast.
Here are some debugging steps you can try when tackling a tricky bug:
- Reproduce the error consistently. If you can’t make it happen, it’s going to be tough to fix it.
- Use logs. It’s like leaving a trail of breadcrumbs to follow if something goes haywire.
- Ask a friend or a colleague to take a look. Sometimes a fresh pair of eyes catches what you’ve missed.
- Don’t be afraid to search online. Someone might have faced the exact same problem.
Lastly, stay updated with the latest debugging trends. It might feel like tech is always evolving (because it is), but keeping up can save you time and effort down the line.
Debugging might never be anyone’s favorite part of coding, but with these tips, it doesn’t have to be a nightmare. Keep calm, code on, and those bugs won’t stand a chance.
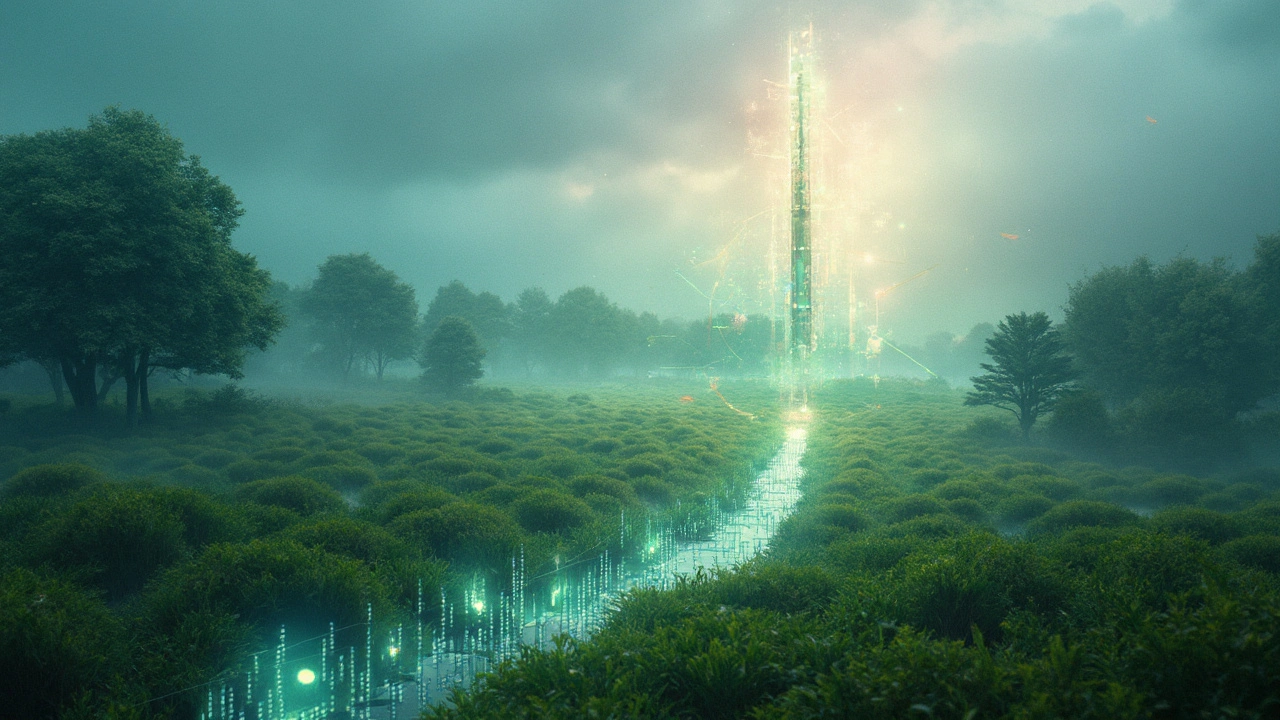
Keeping Up with Trends
The tech world is always on the move. Keeping up with the latest in software development isn't just a perk—it's essential for staying relevant. The coding scene changes faster than your favorite streaming series drops new episodes. So, what's happening in programming tricks.
First off, keep an eye on the most popular programming languages. Today, languages like Python and JavaScript lead the pack because of their versatility and ease of use. Python’s popularity in data science and machine learning can't be ignored, while JavaScript’s dominance on the web remains solid.
AI and machine learning are also important. Everyone's talking about it, and for good reason. Developers leveraging AI to automate boring tasks or even predict bugs before they happen is the cool trend right now. It's all about using smart algorithms to make software smarter.
Don’t forget about the rise of low-code and no-code platforms. They're becoming the go-to for prototype fanatics wanting to whip up apps without getting buried in code. For traditional coders, they're not a replacement but another tool to have in your coding toolkit. They can speed up development times and let you focus on complex problems.
A tip? Follow key influencers and thought leaders in the programming community on platforms like Twitter and LinkedIn. They often share cutting-edge insights. Participating in forums like Stack Overflow can also keep you connected with what's buzzing in the coding realm.
Lastly, think about joining online courses or workshops from sites like Coursera or Udemy. They constantly update material to reflect current trends and innovations. Staying ahead means embracing lifelong learning—it's as simple as signing up and diving into the next lesson. Always remember, in tech, the learning never stops.