Python has earned its stripes as a go-to language for developers worldwide, known for its simple syntax and readability. But beyond the basics, there are intricate tricks that can turn a proficient user into a Python guru. This article dives into these secrets, revealing how to write elegant and effective Python code.
We'll start with understanding the 'Pythonic' way of thinking, a philosophy that emphasizes efficiency and clarity. Moving on, we'll delve into list comprehensions – a Python staple that can make your code not only shorter but more readable. Dynamic typing, a feature that gives Python its flexibility, will be explored next, showing you how to harness its power without stumbling into common pitfalls.
Libraries like NumPy and pandas are game-changers, allowing you to perform complex tasks with ease; we'll uncover how to exploit these tools to their fullest potential. In addition, optimizing code for performance is not just an advanced skill but a necessary art, ensuring your projects run smoothly. Finally, an introduction to effective testing and debugging will round out your skill set, arming you against future coding challenges.
- The Pythonic Way
- List Comprehensions: A Concise Approach
- Dynamic Typing and Its Power
- Harnessing the Magic of Libraries
- Optimization for Performance
- Testing and Debugging Efficiently
The Pythonic Way
Embracing the art of writing 'Pythonic' code is a transformative journey for any developer keen on mastering Python. The term 'Pythonic' refers not just to using Python, but to using it in a way that exploits the unique features and idioms of the language, resulting in code that is both elegant and efficient. At its core, Pythonic code emphasizes readability, a fundamental part of Python's philosophy as stated in the Zen of Python: 'Readability counts.' This principle encourages developers to write code that is clear and straightforward, minimizing the potential for errors and making it easier to understand by others.
Writing in a Pythonic style often involves the use of implicit techniques where Python handles many common tasks automatically. For instance, list comprehensions allow for concise and readable constructions of new list objects, streamlining processes that would be cumbersome and less efficient if performed using traditional loop structures. The Pythonic method values simplicity over complexity, encouraging the coder to select straightforward solutions rather than intricate, ornate ones that could complicate the debugging and maintenance process.
The language’s conventions, such as proper indentation and use of format strings (f-strings), contribute significantly to developing readable code. Python enthusiasts suggest adhering to PEP 8, the style guide for Python code, which promotes consistency within the Python community. This consistency not only makes code more navigable for other users but also enhances collaboration as seemingly distinct pieces of code maintain a uniform appearance and functionality. A Pythonic approach prioritizes leveraging these conventions to maintain the language’s simplicity and directness, which are core tenets of its design philosophy.
Tim Peters, known for the Zen of Python, once communicated: "Simple is better than complex." As developers, this wisdom urges us to declutter our code and adhere to a set of practices that enhances clarity and functionality.
An essential Pythonic technique is the use of Python's dynamic typing and introspection capabilities which allow a programmer to write generic code that works across different types reducing redundancy. Python's dynamic nature also means it supports duck typing—a concept that simplifies code by focusing on what an object can do, rather than what it is. This is a significant stepping stone in achieving efficiency and flexibility, as it allows developers to write functions and classes that seamlessly work with a variety of data types without explicit checks.
Empowering Code with Pythonic Practices
Emphasizing Pythonic practices can also lead to considerable performance improvements, especially through lazy evaluation, such as generator expressions that reduce memory usage by generating items on the fly. Coupling this with Python’s rich set of built-in functions allows developers to maximize efficiency. Functions like map(), filter(), and lambda contribute to a more functional programming style, significantly reducing the need for expansive and often repetitive loops.
Another compelling aspect of Pythonic coding is the emphasis on error handling and robustness, promoted through strategic use of try/except blocks to manage exceptions and ensure the program's flow is not disrupted unexpectedly. Utilizing context managers with the 'with' statement is also advised when dealing with resources such as files, as it ensures they are released properly, adhering to the tenet ‘Explicit is better than implicit’ from the Zen of Python. It's a reminder that clarity should always be a guiding light in every line of code, leading not just to functional, but sustainable and maintainable solutions.
List Comprehensions: A Concise Approach
List comprehensions in Python are a strikingly elegant feature that blends readability with succinctness, transforming loops and map/filter functions into a single, coherent line of code. This Python trick not only enhances code readability but also improves execution speed in many cases. List comprehensions start with an expression followed by a for clause, then zero or more for or if clauses. This flexibility lets you mold complex operations into a compact format that's easy on the eyes but mighty in execution. They're a tool that can, at a glance, redefine multiple lines of code into just one, thereby offering incredible brevity. Yet, before harnessing their full potential, it's important to understand where their real power lies.
Consider a scenario where you have a list of numbers, and you want to create another list containing the squares of these numbers. The traditional approach might involve initializing an empty list and using a for loop to append each squared number. But with list comprehensions, this process becomes downright simple. You can write: [x**2 for x in numbers]
. A single line that captures not just the task but the thought process behind it. In terms of performance, list comprehensions often outperform equivalent loops by optimizing design under the hood, reducing the overhead of function calls, and making the operation native to Python's internals.
The versatility of list comprehensions is further exaggerated by their ability to incorporate conditions and nested arbitrations. Imagine you only want the squares of even numbers. Instead of adding another bulky condition statement, you can introduce an 'if' at the end like this: [x**2 for x in numbers if x % 2 == 0]
. This concise expression avoids clutter and keeps the focus on the logic being implemented. Such clarity isn't just aesthetically pleasing but also a strong point for debugging. Erroneous lines are localized, making tracing errors quicker. An old adage in programming comes to mind: 'Code is more often read than written'. Hence, this trait plays a critical role in collaborative projects.
Despite the brilliance of list comprehensions, they aren't a panacea for all coding challenges. Overusing them can lead to a Python code that's difficult to decipher. A common guideline is to keep the comprehensions simple – avoid nesting and relying on them when the code extends beyond a couple of lines as readability might be compromised, overshadowing the benefits. As the saying by Guido van Rossum, Python's creator, goes:
'Readability counts'.List comprehensions channel this philosophy by balancing clarity with dense coding principles.
Interestingly, Python comprehensions inspire a hierarchy beyond lists. Python extends this paradigm to include set comprehensions, dictionary comprehensions, and even generator expressions. Each holds its own when it comes to providing seamless capabilities to handle diverse data structures effortlessly. By internalizing these tricks, one can elevate coding capabilities significantly, turning heavy everyday tasks into efficient one-liners that not only improve productivity but also maintain a professional grasp on tidy programming techniques.
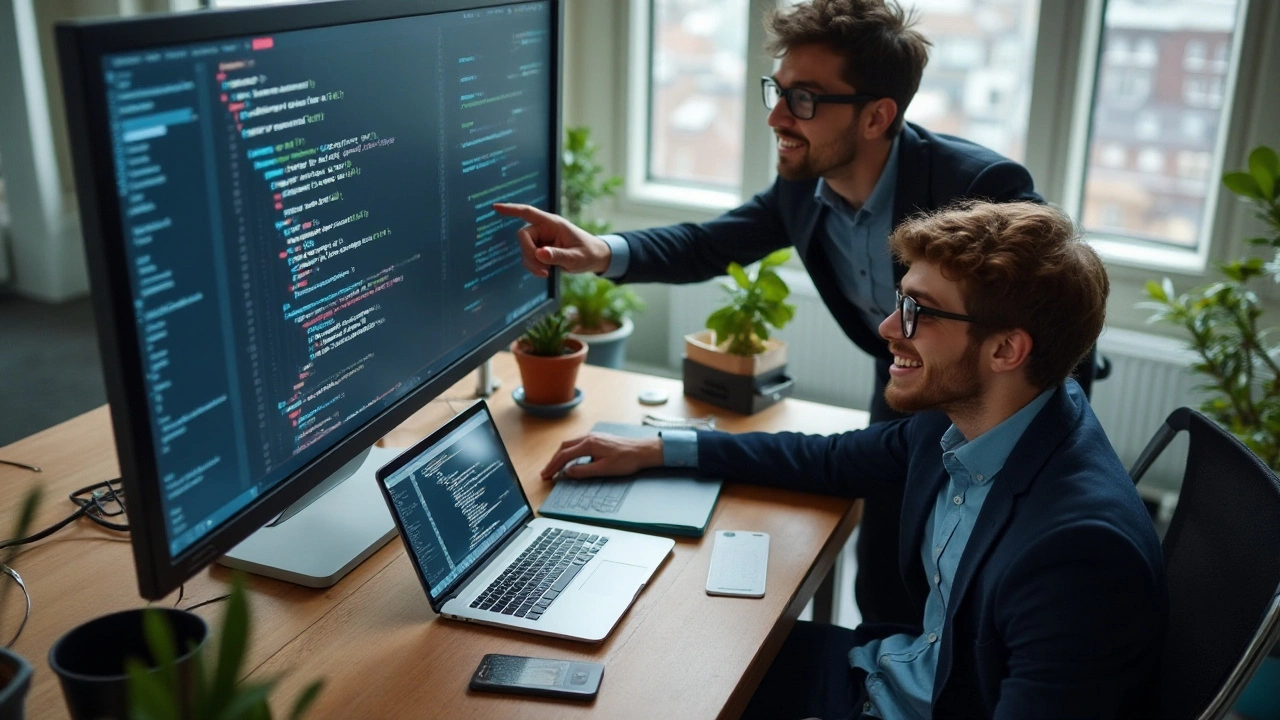
Dynamic Typing and Its Power
Dynamic typing in Python is one of the features that makes the language both incredibly flexible and powerful yet occasionally treacherous for those unprepared. Unlike statically typed languages, Python does not require an explicit declaration of variable types. This means that one can write code more swiftly without tangling in the often cumbersome syntax of type declarations. But with great power comes responsibility—it's crucial to understand how this flexibility works to truly leverage it to your advantage.
To illustrate, consider a situation where you start writing a program that manipulates various data inputs like numbers, strings, and lists, often all within the same function. Python allows you to craft such functions without specifying upfront exactly what type of data they should handle. This leads to more reusable and adaptable code. However, with flexibility also comes ambiguity. When data types can change, bugs can sometimes sneak in, masked by assumptions about a variable's type. Therefore, a strong grasp of dynamic typing involves not just leaving types unspecified, but building robust systems with thoughtful type handling, even if that means using tools like type hinting to clarify intent.
A seasoned developer once remarked,
"Dynamic typing is like poetry in a coding world of prose; it flows, bends, and often surprises, teaching us that rigidity can stifle innovation."Python's dynamic nature often invites a kind of creative problem-solving not found in strictly typed languages. This aspect of Python enhances productivity and the natural flow of coding. Nevertheless, it is advisable to incorporate practices that bring some order to this potential chaos. Employ Python's built-in `type()` function strategically for at-a-glance introspection, or use the `isinstance()` function to ensure type-safe operations where necessary.
But what about performance, you might ask? Isn't dynamic typing slower than static typing? Yes, dynamic typing adds some overhead because types have to be determined at runtime. However, Python offers a suite of optimization techniques, like utilizing the PyPy interpreter or strategically employing Cython for performance-critical sections, thereby mitigating this overhead. Thus, while dynamic typing presents certain performance challenges, its benefits in speed of development and ease of use often far outweigh these concerns.
Embracing dynamic typing doesn’t imply forgoing the benefits of clarity and error prevention that types can offer. Python’s flexibility extends to allowing developers to incorporate type hints as a part of its syntax, enhancing readability and maintainability without sacrificing the dynamism that makes the language so beloved. The power of dynamic typing lies not just in the freedom it offers but also in learning to combine that freedom with Python's robust toolset for type management to create clean, efficient, and powerful code.
In essence, mastering dynamic typing in Python involves more than simply riding its wave of flexibility. It requires a nuanced approach to leverage the liberty it gives while maintaining a vigilant eye for potential pitfalls—transforming you into a true Python guru. So, whether you're crossing data types to craft elegant solutions or wrapping your head around Python's inferential prowess, understanding dynamic typing's artisanal blend of freedom and control might just be key to unlocking your full potential in this beloved language.
Harnessing the Magic of Libraries
Python's extensive library ecosystem is one of its standout features, providing powerful tools to tackle everything from data manipulation to machine learning. These libraries, like NumPy, pandas, and SciPy, serve as building blocks that can accelerate both learning and application development. Integrating them effectively into your projects can transform simple scripts into robust applications. Understanding how to choose and use the right libraries is akin to possessing a secret skill. For instance, NumPy excels in handling multi-dimensional arrays and performing mathematical operations, which are vital in data processing tasks. This library significantly reduces the complexity of your code, thereby speeding up the development process. Moreover, its integration with other libraries enhances both flexibility and functionality.
The pandas library stands out for its ability to handle and manipulate data with ease, functioning similarly to a Swiss Army knife for any data scientist. With pandas, tasks like filtering, grouping, and merging data become intuitive. It's not just about its capabilities but the efficiency it brings to data handling. Leveraging pandas in your Python scripts allows you to focus on the bigger picture, drawing insights from data rather than getting bogged down in coding minutiae.
"The beauty of pandas is its simplicity; its power lies not in what it can do, but in how easily it allows you to do complex tasks." - A Data Scientist's Guide.Each library, with its unique features, offers ways to streamline your workflow. Emphasizing this makes Python one of the most versatile programming languages available.
Another library worth mentioning is SciPy, particularly for those entrenched in scientific and technical computing. SciPy builds on NumPy's capabilities, offering modules for optimization, integration, and Fourier transformations, which are crucial for engineering and scientific tasks. Such libraries minimize the need for complex algorithms from scratch, saving both time and effort. These tools support the iterations and modifications common in scientific research projects, allowing for rapid testing and validation of hypotheses. As you delve deeper into these libraries, a world of possibilities opens up, letting you tackle increasingly sophisticated projects with confidence.
For machine learning enthusiasts, libraries like TensorFlow and Keras make implementing AI models more approachable. TensorFlow, developed by Google Brain, is especially popular for its ability to run computations on CPUs and GPUs, enhancing performance while training complex models. Complementing TensorFlow, Keras provides a user-friendly API to build neural networks, making it ideal for quick prototyping and results validation. These libraries have democratized machine learning, enabling even beginners to delve into fields previously dominated by experts.
By investing time in mastering these libraries, you're essentially enhancing your toolkit, allowing you to take advantage of Python's full potential. The time saved in efficiently leveraging these tools can be redirected towards developing innovative solutions. This is why knowing not just Python but also its libraries makes a difference between a programmer and a guru.
Library | Use Case |
---|---|
NumPy | Mathematical operations on arrays |
pandas | Data manipulation and analysis |
SciPy | Scientific computing tasks |
TensorFlow | Machine learning models |
Keras | User-friendly neural network API |
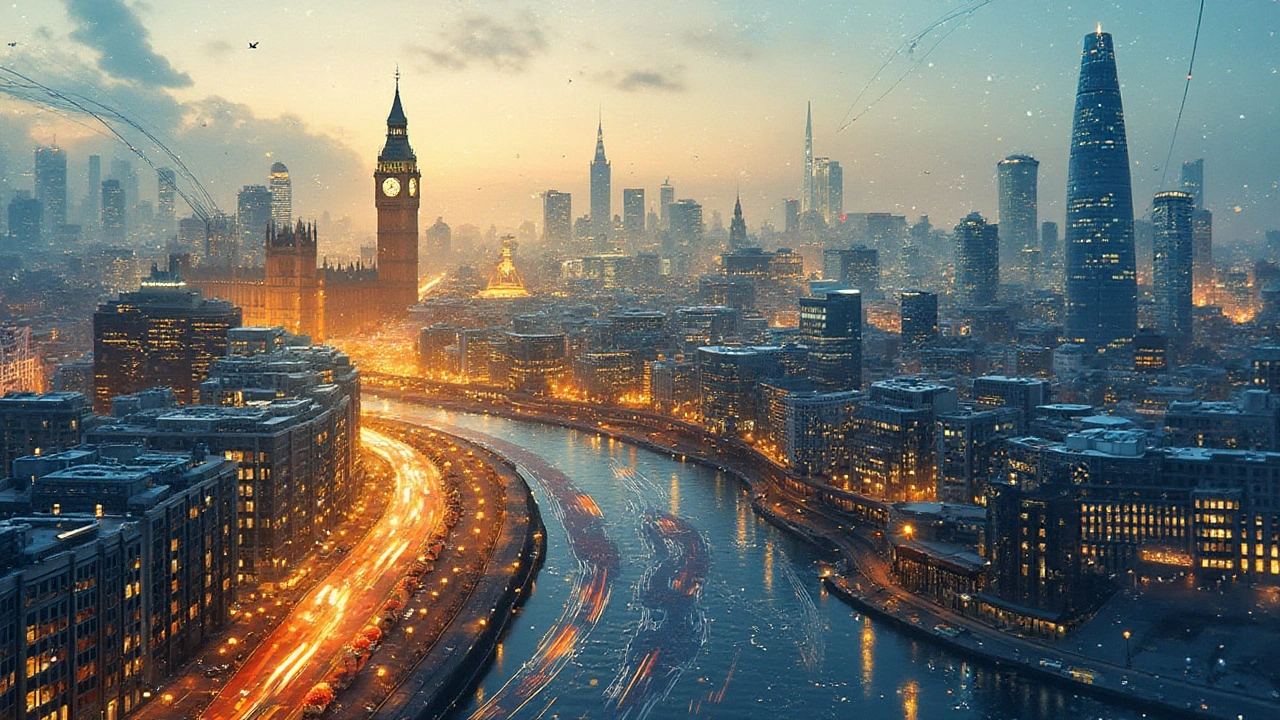
Optimization for Performance
When it comes to crafting a Python application that truly sings, understanding how to optimize performance is key. Many developers often overlook the importance of efficient coding once they get things to work. However, performance optimization can make the difference between a program that merely functions and one that impresses with its speed and responsiveness. This section will explore strategies to refine your code, helping you achieve the nimble efficiency that is characteristic of expert developers.
One fundamental tip is to profile your code before making changes. It might sound obvious, but knowing where the bottlenecks are is crucial. Python provides tools such as cProfile and timeit, which can help identify slow parts of your code. Start by focusing your optimization efforts on these areas, since premature optimization is often the root of unnecessary complexity. Once you make these pinpointed changes, you'll want to profile again to ensure that new bottlenecks aren't created.
Memory management often goes hand in hand with optimizing for performance. Inefficient use of memory can slow down your program considerably. Utilize generators instead of lists wherever applicable, as they are less memory-intensive. Generators supply their elements one at a time and only when required. This lazy evaluation means you won't allocate memory for the entire data set at once, significantly improving performance. This practice becomes even more vital in data-heavy applications where traditional lists might lead to memory exhaustion.
Utilizing the right data structures can have a profound impact on both memory efficiency and execution speed. Python offers diverse built-in types such as lists, sets, and dictionaries. For instance, if your primary concern is fast membership tests, sets and dictionaries are the go-to options due to their underlying hash table implementations. Algorithms that find subsets or unions will benefit substantially from the aforementioned data structures, thanks to their average O(1) lookup time for elements.
Consider leveraging Python's multi-threading features in specific scenarios. Though the Global Interpreter Lock (GIL) restricts threads in executing Python bytecodes concurrently, threading is still effective for I/O-bound operations. On the other hand, for CPU-bound tasks, the multiprocessing module is effective, as it bypasses the GIL by executing subprocesses that have independent Python interpreters.
And this advice from the legendary Donald Knuth always resonates:
“The real problem is that programmers have spent far too much time worrying about efficiency in the wrong places and at the wrong times; premature optimization is the root of all evil.”This wisdom underscores the need for strategic and well-considered optimization.
Finally, the use of native code via C extensions or employing tools like Cython can produce a drastic performance boost. Cython compiles Python code into C, and with minor modifications, you can attain near-native performance for critical portions of your codebase. When executed wisely, these strategies transform Python applications, enabling them to handle high-load situations gracefully and maintain responsiveness.
Testing and Debugging Efficiently
To become a true Python guru, mastering the art of testing and debugging is imperative. This process is akin to detective work, where developers meticulously comb through code to ensure everything functions as intended. Python tips for efficient debugging often begin with understanding common error messages and knowing where to look first when issues arise. Start by examining traceback logs carefully; they hold the key to deciphering where your Python program might have gone awry. Building a habit of regularly writing tests can significantly shield you from unpleasant surprises down the road. Unit and integration tests ensure each part of your program, and their interactions work as expected before deployment. With tools like PyTest, testing becomes less of a chore and more of a standard practice, reinforcing the code's robustness.
Seasoned developers often advise against testing and debugging after the fact. Instead, adopt a test-driven development (TDD) approach where you write tests before the actual creation of the feature. It might seem counterintuitive at first, but TDD can lead to cleaner and more reliable outcomes, encouraging forethought in coding. Using version control tools like Git combined with continuous integration pipelines can provide real-time feedback, catching errors early in the process. This practice is akin to having a safety net, preventing small mistakes from snowballing into significant problems later. As coding tricks go, adopting a structured debugging routine can dramatically improve the efficiency of your workflow.
Let's take a moment to appreciate the ecosystem of debugging tools available in the Python realm. The Python Debugger (PDB) is a powerful, built-in tool that allows you to inspect running code, set breakpoints, and step through code execution one line at a time. This micro view of your application gives you the precision needed to pinpoint exactly where things go wrong. Third-party tools like the IPython shell offer enhanced functionality over the standard interactive Python shell, enabling dynamic exploration of your code with intuitive commands. Making this part of your regular development diet ensures that you can swiftly tackle bugs with precision and learn from the mistakes along the way.
For an added layer of assurance, logging can be your silent assistant in the journey of debugging. By recording events that happen during the runtime of your program, logging helps trace down the root of the issues by creating narratives of your application's execution flow. Imagine it as a personal diary of your program, recording its every step and hiccup, helping you spot where things go wrong. According to seasoned developers like Paul Graham, "Great debugging skills are often the key differentiator between good and great programmers."
"Great debugging skills are often the key differentiator between good and great programmers." — Paul GrahamEffective use of logs can save hours of head-scratching and head-desk moments by offering clues and insights into the inner workings of your software.
Numerous Python Python skills can further assist in this endeavor. Handling exceptions gracefully with try-catch blocks will not only prevent your program from unceremoniously crashing but also provide user-friendly outputs that maintain the application's credibility. Implementing useful debug messages within these blocks equips you with quick insights without the need to comb through entire codebases. This actionable information can lead to quicker resolutions. Moreover, including conditional breakpoints can prove invaluable, stopping execution not just anywhere, but right where a particular condition is met. This pinpoint accuracy cuts down on the time you spend on trial and error, making your debugging process significantly more efficient.
Coding tricks extend to collaborative efforts as well, making pair debugging a beneficial practice, especially for complex problems. Sometimes a fresh pair of eyes can spot what you might have missed. Additionally, rubber duck debugging, a humorous yet effective method, involves explaining your code and logic to an inanimate object. The simple act of articulating your thought process can often reveal the solution, or at least a clearer path to finding it, which you may have overlooked initially. As you refine these skills, you'll find yourself not only solving problems more quickly but also deepening your understanding of Python as a language, leaving you well-prepared for any coding challenge that comes your way.