Python remains one of the most beloved programming languages across the globe, admired for its simplicity and versatility. Whether you are just dipping your toes in the programming waters or you're a seasoned developer, mastering Python's nuances can offer a significant edge.
In this guide, we'll dive into some lesser-known but incredibly useful tricks that can take your Python programming skills to the next level. Think of this as a conversation between friends who share a passion for coding and wish to learn new hacks that could simplify their lives. From improving your coding efficiency with list comprehensions to unleashing the power of decorators, there's always something new to discover in Python.
By the end of this article, you'll have a new arsenal of Python tricks to solve real-world problems more effectively and even impress your peers. So, get comfy, perhaps with a fluffy companion by your side, and let's unravel the magic of Python programming together.
- Understanding Python's Flexibility
- Efficient Coding With List Comprehensions
- Magic of Python Decorators
- Debugging Like a Pro
- Automating Tasks and Beyond
Understanding Python's Flexibility
When it comes to programming, flexibility is a key trait that makes learning not just easier but also more enjoyable. Python excels in this arena with its adaptable nature, seamlessly accommodating both simple scripts and large-scale applications. This inherent flexibility stems from Python's philosophy of readability and simplicity, making it the choice language for developers who thrive on creating diverse solutions with fewer lines of code. With Python, you can switch from building a web application to automating tedious tasks without breaking a sweat. This agile transition is possible due to Python's vast collection of libraries and frameworks, providing tools for every need imaginable.
One of the standout features of Python is its dynamic typing, which means you can write quick prototypes without getting bogged down in type declarations. This agility speeds up the development process, allowing for rapid iteration and experimentation. However, this doesn’t mean a lack of control; with type hints introduced in Python 3.5, you can have the best of both worlds—flexibility when you need it and structure when complexity creeps in. As an interpreted language, Python also lets you test code in real-time, contributing to a smoother development process.
"Python's readability and simplicity of syntax make it the easiest choice for developers who want to focus on problem-solving rather than focusing on deciphering code." — Guido van Rossum, Python's creator.
Python's extensibility with existing libraries and APIs makes it a playground for innovators. Whether you're diving into data analysis with Pandas, exploring artificial intelligence with TensorFlow, or developing games using Pygame, Python offers the flexibility to create without limits. Its community-driven nature means there is ample support and a rich repository of resources just a search away. Moreover, Python's compatibility with other languages lets developers use it alongside C++, Java, and more, facilitating a truly polyglot approach to software creation.
The robustness of Python's ecosystem also contributes to its flexibility. The language's package manager, pip, stands out as a powerful tool that streamlines the installation of countless third-party modules. With pip, extending your project with external libraries is as simple as a command line entery. For instance, adding a library like Beautiful Soup for web scraping, Flask for microservices, or PyQt for GUI development has never been easier. Python's ongoing evolution, backed by an active community, ensures it stays at the forefront of modern programming languages.
Python programming is more than just a coding exercise; it’s a way to think about and solve problems. Its flexibility fosters an environment where creativity and technical prowess can collaborate seamlessly. With these attributes, learning Python might just be the most rewarding step you take in your programming journey, preparing you to navigate diverse project requirements with ease. Embrace Python’s inherent flexibility, and the opportunities to innovate are boundless.
Efficient Coding With List Comprehensions
List comprehensions in Python programming are an elegant way to create lists. For those diving into the realm of code, understanding this feature is quintessential. It combines the power and simplicity of Python and provides a concise way to generate a list by writing a single line of code. Unlike traditional for loops, list comprehensions are easier to write and often more readable, especially for small, simple operations. Imagine cutting down several lines of verbose code into just one – it's a small victory every coder needs. This feature not only saves time but can also prevent errors, as there are fewer moving parts to a simpler implementation.
Developers often use list comprehensions for tasks such as filtering elements, mapping functions, or even multiplying elements in the list by a certain number – all neatly done in a single line. Consider the scenario where you need a list of squares from numbers 1 through 10. Instead of setting up a for loop, a list comprehension could accomplish this with a straightforward mathematical expression embedded in the creation process.
How to Use List Comprehensions
The beauty in list comprehensions lies in their syntax, which is smoother and more Pythonic. The basic structure includes brackets containing an expression followed by a for clause, then zero or more for or if clauses. The result is a new list resulting from evaluating the expression in the context of the for and if clauses that follow it. Let’s translate this into action:
- Create structured lists: With list comprehensions, transforming collections based on existing data becomes a breeze. Whether you want to strip whitespace, filter out unwanted characters, or extract specific features from strings, you can do it with elegance and precision.
- Efficient data processing: By minimizing the overhead of loops and append operations, list comprehensions tend to be faster when working with large data sets. You'll find this particularly beneficial when working on performance-critical applications or data-intensive operations.
- Avoid unnecessary complexity: Readability is an essential aspect of good code, and list comprehensions support this by reducing boilerplate code. It demonstrates Python’s philosophy of simplicity and beauty in code.
"Code is like humor. When you have to explain it, it’s bad." – Cory House
This quote perfectly encapsulates why list comprehensions are a preferred choice. They help keep your code clean, clear, and concise, thus easing the cognitive load on others who might need to maintain or review your work down the road. So, next time you find yourself pondering over a multi-line loop to create a list, pause, and consider if a list comprehension might be your answer. You’ll not only improve your code efficiency but also gain an admiration for Python's ability to make life just a tad bit easier.
The concept ties into more than just syntax; it's about enhancing the programming ethos: less complexity, more clarity. As you embrace the discipline of list comprehensions, you'll be joining ranks with experienced programmers who have discovered this nifty trick and never looked back. Whether you're handling numbers, strings, or complex objects, the potential unlocked by list comprehensions inspires a smoother, smarter workflow.
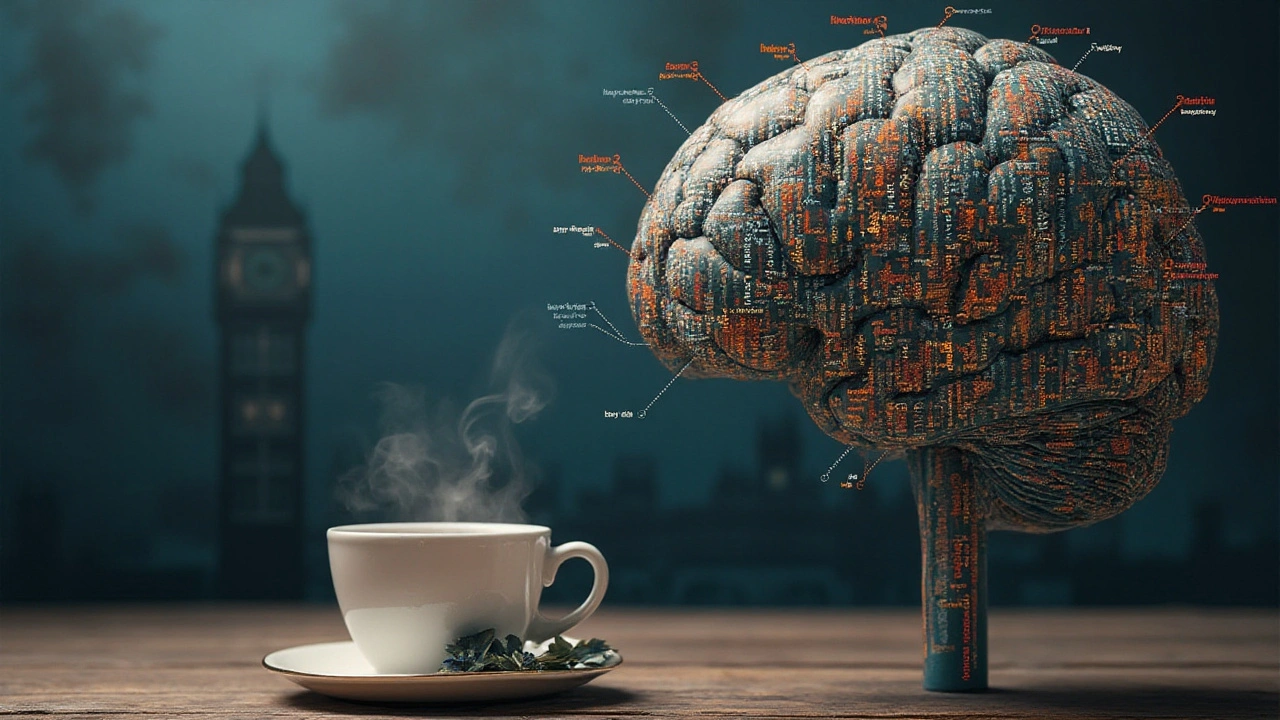
Magic of Python Decorators
Decorators in Python programming are like the sprinkles on a cupcake, enhancing function definitions with additional capabilities without altering their core. They hold a remarkable place in Python as a design pattern that allows programmers to modify the behavior of a function or a class. Imagine having the power to extend or modify the capabilities of functions you wrote, providing a clean and elegant solution for logging, enforcing access control, instrumentation, and much more. This dynamic quality is what makes decorators an intriguing feature of the language. Python decorators are implemented using a '@' symbol before a function they decorate, which not only saves lines of code but also makes the code more readable.
The concept of decorators lies in the notion that functions in Python are first-class citizens. They can be passed around as arguments, used as return values, and even defined within other functions. This flexibility allows decorators to be as much about wrapping functions with additional behavior as they are about transformation. For instance, a common use case of decorators is in web development frameworks where authentication is crucial. A decorator can validate a user's credential for accessing specific views without cluttering the main logic. This not only streamlines the coding process but also separates concerns effectively.
Let's delve deeper into how you can create a simple decorator in Python. The basic structure involves defining a function, say 'logger', which takes another function 'func' as its only parameter. Within 'logger', a nested function 'wrapper' is defined that calls 'func'. The 'wrapper' function can additionally print logs or perform any additional task before or after calling 'func'. Finally, 'logger' returns this 'wrapper' function. When this 'logger' is applied to a target function using the '@' symbol, you get the same functionality as before, but now it's enriched with logging capability. Consider that decorators truly leverage Python's dynamic nature, turning code maintenance into a less daunting task.
Many respected platforms acknowledge the utility of decorators. According to a Python expert in 'Real Python', "Decorators abstract away common logic or boilerplate code, while also allowing the main concern to shine through in your code." The role of decorators in efficient coding and maintaining clarity is worth noting. It's not just about adding functionality but doing so in a way that cleanly separates business logic from auxiliary operations. This makes your Python scripts not only more robust but also easier to understand and manage over time.
If you're yet to embrace decorators fully, consider starting with simple scenarios like timing functions to gauge performance. Python decorators can be your ticket to writing more concise and effective code, leading to a smoother coding experience overall. Remember, like all coding tricks, the key is to practice and experiment. Play around with decorators by creating custom ones — they can be a fun way to unlock new coding possibilities you might not have considered before!
Decorator Use Case | Example |
---|---|
Logging | Enhance functions by automatically logging their usage. |
Authorization | Restrict access to functions based on user roles. |
Caching | Store expensive function outputs for later use. |
Debugging Like a Pro
Delving into the world of Python programming can be exciting, but even the most seasoned programmers encounter pesky bugs that disrupt their workflow. Fear not, though, because honing your Python programming debugging skills is not only achievable but life-changing for your coding projects. Let’s unravel some techniques that will transform your debugging capabilities so you can code with confidence and competence.
To kick things off, let's discuss the beauty of the built-in `pdb` (Python Debugger) module, a tool every developer should have in their toolbox. Utilizing `pdb` allows you to set breakpoints, inspect stack traces, and interactively explore variables within the current scope. It's akin to stepping inside your code and having a chat with it, unraveling where things might have gone astray. But the trick is not only in knowing `pdb` exists but also mastering its commands like `next`, `continue`, and `list`, each strategically guiding you through your program’s lifecycle as you hunt down elusive bugs.
The art of logging is another vital skill, involving strategically placing logs throughout your code. Libraries like Python’s `logging` module provide granular control over what messages you want to record and how they should be displayed. From `DEBUG` to `CRITICAL`, understanding the range of severity levels allows developers to create informative logs that narrate their program’s journey, highlighting potential points of failure. By designing logs mindful of your program’s complexity, you reduce the guesswork considerably, painting a clearer picture of your code’s behavior.
Moreover, a sophisticated yet simple trick involves using assertions. An assertion functions as a sanity check within your code. By using the `assert` statement, you can validate assumptions made within a code segment. For instance, if certain conditions must hold true for your program to execute correctly, employing an assertion statement lets you verify this without manually sifting through line after line of code.
A great programmer once said, "The sooner you start to code, the longer the program will take." Implementing rigorous testing and debugging practices stands as testament to the insight within these words.
Another strategy involves leveraging Python’s powerful exception handling mechanisms. Missteps in handling exceptions often lead to rampant bugs. However, with thoughtful exception handling, you clarify the catch blocks and manage various exceptions without letting errors slip past unnoticed. It’s worthwhile to remember that catching exceptions should be specific, allowing for insightful error messages that guide rather than confuse.
Finally, don't underestimate the power of peer reviews and collaboration. Inviting a fellow developer to review your code can often reveal insights and uncover bugs that you might overlook. Fresh eyes can catch details that escape even the most meticulous coders, and collaborative debugging often fosters a learning environment where knowledge and skills are shared and developed collaboratively.
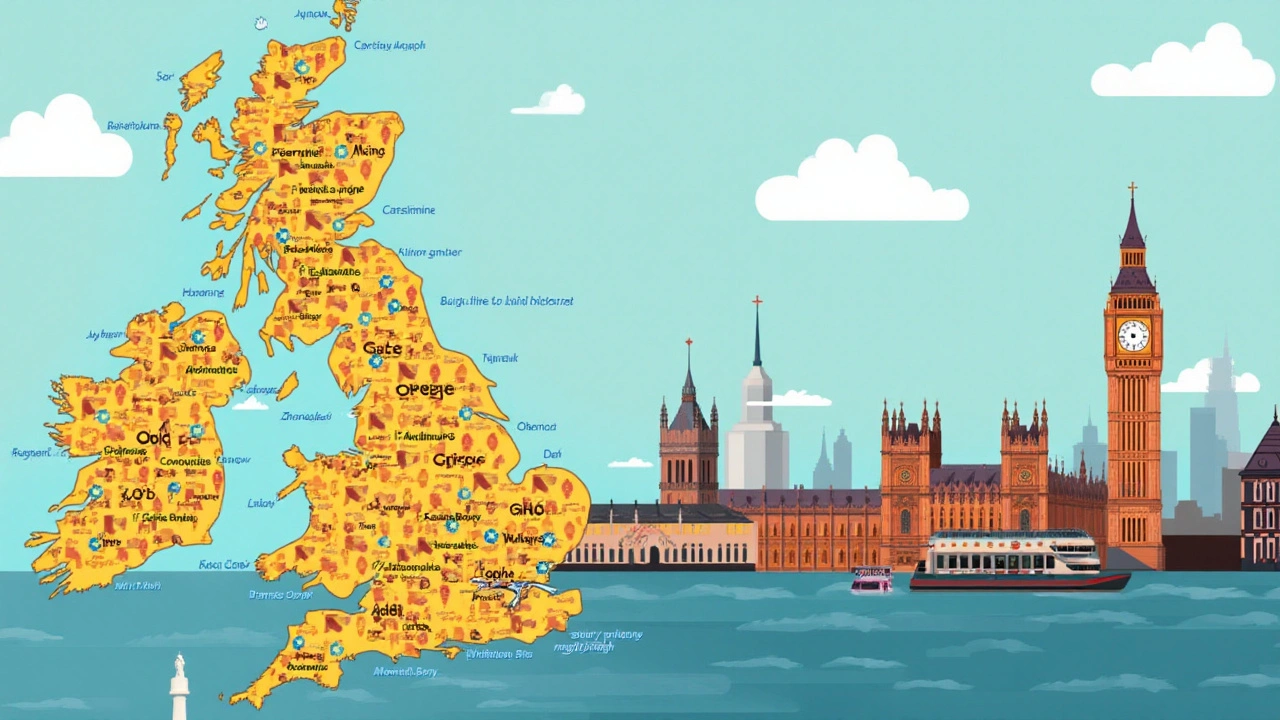
Automating Tasks and Beyond
When it comes to harnessing the power of Python programming, automating repetitive tasks often tops the list of needs for both novices and professionals alike. Python's extensive library and easy syntax make it especially suitable for automation, transforming mundane chores into streamlined processes, thereby saving considerable time and effort. At this juncture, it’s worthwhile to highlight Python's strength in utilizing modules such as 'os', 'shutil', and 'glob'. These are tailored to interact with the operating system, allowing developers to manage files and directories with ease. Whether it’s renaming a batch of files or moving directories, Python provides the tools needed to get the job done flawlessly. The 'os' module helps in accessing operating system interfaces, which is where automation begins. By mastering these modules, you can create scripts that handle large volumes of data without lifting a finger beyond typing the initial script.
Automating goes beyond just saving time. It opens up limitless possibilities in every sector, from data scraping and report generation to scheduled email dispatching and data entry. By leveraging libraries like 'selenium', developers can even automate web browser interactions, a boon for tasks such as web scraping or automated testing. Consider creating a script to log into your favorite social media account, post updates, or gather data from your network. This blend of creativity and problem-solving showcases Python’s versatility when it comes to tangible real-world applications. In fact, renowned tech advocate Tim Peters once said,
"Python is about having the right data structures and the right algorithms to solve your problems."This philosophy embodies the essence of task automation — using Python to conquer challenges by working smarter, not harder.
Moreover, employing Python for automation is not the exclusive domain of large enterprises. Small businesses and individuals stand to gain just as much from its advantages. Imagine a small online retail business utilizing Python scripts to keep inventory updated across multiple platforms or automating backend data entry processes. This can free up valuable resources, hidden under the piles of routine tasks, allowing focus to shift toward core business development. Such a resource allocation is invaluable, be it for a solo entrepreneur or a sprawling enterprise. For anyone looking to dive deeper into automating with Python, it's wise to explore packages like 'requests' for handling web requests and 'pandas' for data manipulation. These tools, combined, position Python as a powerhouse in managing and manipulating large datasets efficiently and effectively.
The future of automation in Python shows promise too, especially when one considers emerging fields such as artificial intelligence and machine learning, where automation plays a critical role. Python's simplicity allows beginners and experts alike to focus more on solving complex problems rather than getting bogged down by the intricacies of the syntax. By staying updated with Python’s evolving libraries and tools, developers can continue to innovate and push the boundaries of what automation can achieve. Breakthroughs in science, finance, healthcare, and many other fields rely on automating complex processes, and Python remains a steadfast ally in breaking new ground. As a guiding principle, always begin with identifying repetitive tasks in your workflow, and then explore how Python can be employed to reduce or eliminate them.